XmlDataProvider and XmlNamespaceMapping
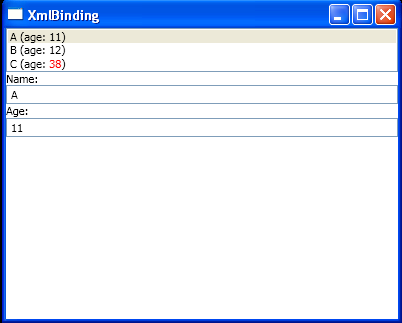
<Window x:Class="XmlBinding.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:XmlBinding"
Title="XmlBinding" Height="325" Width="400">
<Window.Resources>
<XmlDataProvider x:Key="Company" XPath="/sb:Company/sb:Employee">
<XmlDataProvider.XmlNamespaceManager>
<XmlNamespaceMappingCollection>
<XmlNamespaceMapping Uri="http://company.com" Prefix="sb" />
</XmlNamespaceMappingCollection>
</XmlDataProvider.XmlNamespaceManager>
<x:XData>
<Company xmlns="http://company.com">
<Employee Name="A" Age="11" />
<Employee Name="B" Age="12" />
<Employee Name="C" Age="38" />
</Company>
</x:XData>
</XmlDataProvider>
<local:AgeToForegroundConverter x:Key="ageConverter" />
</Window.Resources>
<StackPanel DataContext="{StaticResource Company}">
<ListBox ItemsSource="{Binding}" IsSynchronizedWithCurrentItem="True">
<ListBox.GroupStyle>
<x:Static Member="GroupStyle.Default" />
</ListBox.GroupStyle>
<ListBox.ItemTemplate>
<DataTemplate>
<TextBlock>
<TextBlock Text="{Binding XPath=@Name}" />
(age: <TextBlock Text="{Binding XPath=@Age}" Foreground="{Binding XPath=@Age, Converter={StaticResource ageConverter}}" />)
</TextBlock>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
<TextBlock>Name:</TextBlock>
<TextBox Text="{Binding XPath=@Name}" />
<TextBlock>Age:</TextBlock>
<TextBox Text="{Binding XPath=@Age}" Foreground="{Binding XPath=@Age, Converter={StaticResource ageConverter}}" />
</StackPanel>
</Window>
//File:Window.xaml.cs
using System;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Shapes;
using System.Collections.Generic;
using System.Diagnostics;
using System.Collections;
using System.Data;
using System.Data.OleDb;
using System.ComponentModel;
using System.Xml;
namespace XmlBinding {
public partial class Window1 : Window {
public Window1() {
InitializeComponent();
}
ICollectionView GetCompanyView() {
DataSourceProvider provider = (DataSourceProvider)this.FindResource("Company");
return CollectionViewSource.GetDefaultView(provider.Data);
}
}
public class AgeToForegroundConverter : IValueConverter {
public object Convert(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture) {
Debug.Assert(targetType == typeof(Brush));
int age = int.Parse(value.ToString());
return (age > 25 ? Brushes.Red : Brushes.Black);
}
public object ConvertBack(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture) {
throw new NotImplementedException();
}
}
}
Related examples in the same category