Define friend function for <<
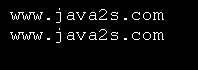
#include <iostream>
#include <cstring>
#include <cstdlib>
using namespace std;
class StringClass {
char *p;
int len;
public:
StringClass(char *ptr);
~StringClass() {
delete [] p;
}
friend ostream &operator<<(ostream &stream, StringClass &ob);
};
StringClass::StringClass(char *ptr)
{
len = strlen(ptr)+1;
p = new char [len];
if(!p) {
cout << "Allocation error\n";
exit(1);
}
strcpy(p, ptr);
}
ostream &operator<<(ostream &stream, StringClass &ob)
{
stream << ob.p;
return stream;
}
int main()
{
StringClass stringObject1("www.java2s.com"), stringObject2("www.java2s.com");
cout << stringObject1;
cout << endl << stringObject2 << endl;
return 0;
}
Related examples in the same category