Private and protected member variables
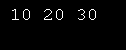
#include <iostream>
using namespace std;
class MyClass {
int a; // private by default
protected: // still private relative to MyClass
int b;
public:
int c;
MyClass(int n, int m) {
a = n;
b = m;
}
int geta() {
return a;
}
int getb() {
return b;
}
};
int main()
{
MyClass object(10, 20);
object.c = 30; // OK, c is public
cout << object.geta() << ' ';
cout << object.getb() << ' ' << object.c << '\n';
return 0;
}
Related examples in the same category