Use a map of strings to create a phone directory.
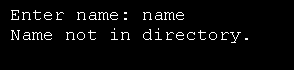
#include <iostream>
#include <map>
#include <string>
using namespace std;
int main()
{
map<string, string> directory;
directory.insert(pair<string, string>("T", "4444"));
directory.insert(pair<string, string>("C", "9999"));
directory.insert(pair<string, string>("J", "8888"));
directory.insert(pair<string, string>("R", "0000"));
string s;
cout << "Enter name: ";
cin >> s;
map<string, string>::iterator p;
p = directory.find(s);
if(p != directory.end())
cout << "Phone number: " << p->second;
else
cout << "Name not in directory.\n";
return 0;
}
Related examples in the same category