Using a multiset to record responses to a polling question.
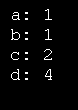
#include <iostream>
#include <set>
using namespace std;
enum response { a,
b,
c,
d,
e };
int main() {
multiset<response> question;
// create some responses
question.insert(response(a));
question.insert(response(b));
question.insert(response(c));
question.insert(response(c));
question.insert(response(d));
question.insert(response(d));
question.insert(response(d));
question.insert(response(d));
// display results
cout << "a: ";
cout << question.count(a) << endl;
cout << "b: ";
cout << question.count(b) << endl;
cout << "c: ";
cout << question.count(c) << endl;
cout << "d: ";
cout << question.count(d) << endl;
return 0;
}
Related examples in the same category