Use string: find, string::npos
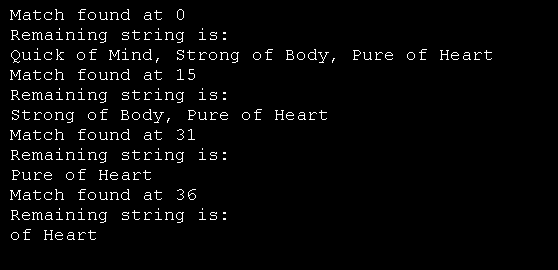
#include <iostream>
#include <string>
using namespace std;
int main()
{
int i;
string s1 = "Quick of Mind, Strong of Body, Pure of Heart";
string s2;
i = s1.find("Quick");
if(i != string::npos) {
cout << "Match found at " << i << endl;
cout << "Remaining string is:\n";
s2.assign(s1, i, s1.size());
cout << s2;
}
cout << endl;
i = s1.find("Strong");
if(i != string::npos) {
cout << "Match found at " << i << endl;
cout << "Remaining string is:\n";
s2.assign(s1, i, s1.size());
cout << s2;
}
cout << endl;
i = s1.find("Pure");
if(i!=string::npos) {
cout << "Match found at " << i << endl;
cout << "Remaining string is:\n";
s2.assign(s1, i, s1.size());
cout << s2;
}
cout << endl;
// find list "of"
i = s1.rfind("of");
if(i!=string::npos) {
cout << "Match found at " << i << endl;
cout << "Remaining string is:\n";
s2.assign(s1, i, s1.size());
cout << s2;
}
return 0;
}
Related examples in the same category
1. | String type class | | 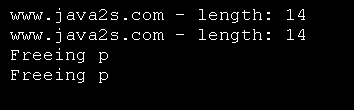 |
2. | A filter to remove white-space characters at the ends of lines. | |  |
3. | A string demonstration: assignment, concatenate, compare | | 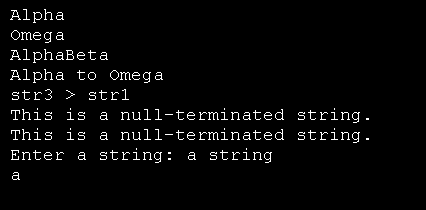 |
4. | Demonstrate insert(), erase(), and replace(). | | 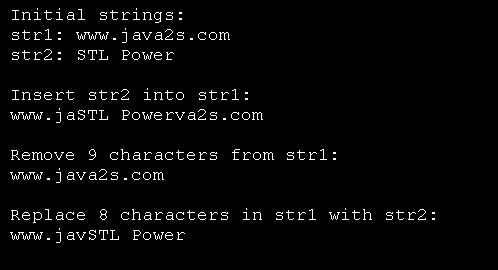 |
5. | Strings: size, iterator, count, begin and end | | 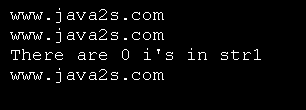 |
6. | string: find( ) and rfind( ) | | 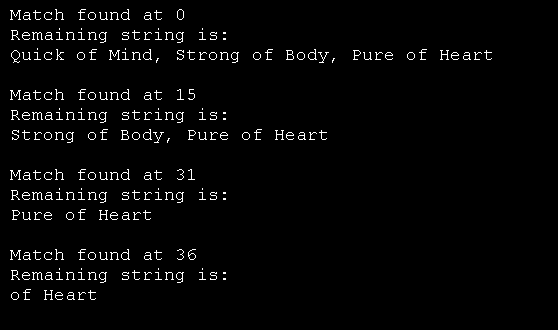 |
7. | Print a name in two different formats | |  |
8. | Several string operations: substr | | 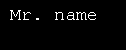 |
9. | String Char Indexing | |  |
10. | String Find and replace | |  |
11. | String Size | |  |
12. | String SizeOf | | 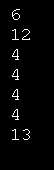 |
13. | A short string demonstration | | 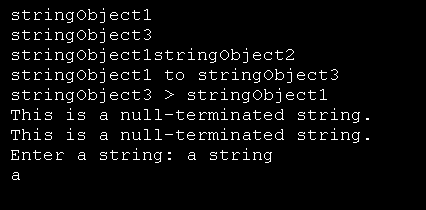 |
14. | String insert(), erase(), and replace() | | 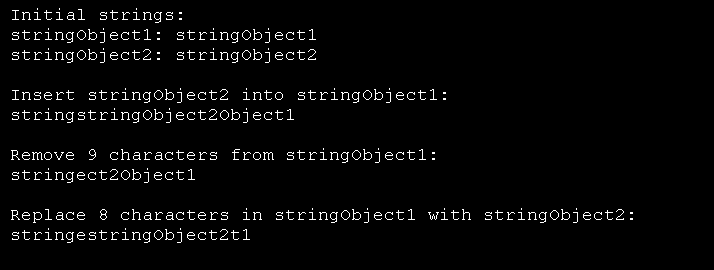 |
15. | string variable instead of a character array | | 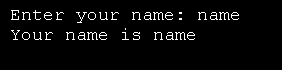 |
16. | Inputting Multiple Words into a String | | 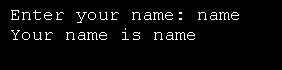 |
17. | Adding Strings | |  |
18. | Read string from console | | 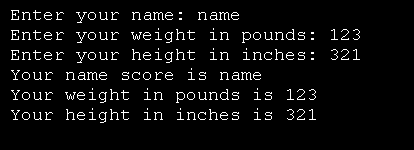 |
19. | Insert, search, and replace in strings. | | 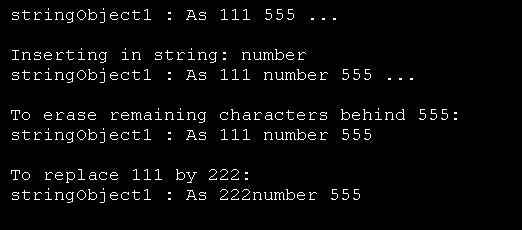 |
20. | Accessing Characters In Strings | |  |