Demonstrate peek() in ifstream
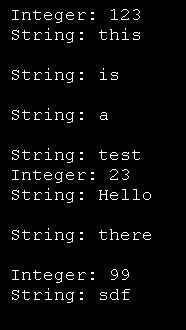
#include <iostream>
#include <fstream>
#include <cctype>
using namespace std;
int main()
{
char ch;
ofstream out("test", ios::out | ios::binary);
if(!out) {
cout << "Cannot open output file.\n";
return 1;
}
char str[80], *p;
out << 123 << "this is a test" << 23;
out << "Hello there!" << 99 << "sdf" << endl;
out.close();
ifstream in("test", ios::in | ios::binary);
if(!in) {
cout << "Cannot open input file.\n";
return 1;
}
do {
p = str;
ch = in.peek();
if(isdigit(ch)) {
while(isdigit(*p=in.get()))
p++;
in.putback(*p);
*p = '\0';
cout << "Integer: " << atoi(str);
}
else if(isalpha(ch)) {
while(isalpha(*p=in.get())) p++;
in.putback(*p);
*p = '\0';
cout << "String: " << str;
}
else
in.get();
cout << '\n';
} while(!in.eof());
in.close();
return 0;
}
Related examples in the same category