Throwing an exception from a function
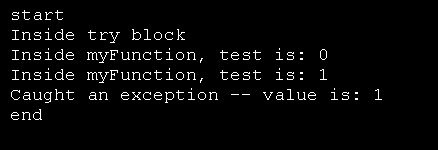
#include <iostream>
using namespace std;
void myFunction(int test)
{
cout << "Inside myFunction, test is: " << test << endl;
if(test) throw test;
}
int main()
{
cout << "start\n";
try { // start a try block
cout << "Inside try block\n";
myFunction(0);
myFunction(1);
myFunction(2);
} catch (int i) { // catch an error
cout << "Caught an exception -- value is: ";
cout << i << endl;
}
cout << "end";
return 0;
}
Related examples in the same category