Another Demo: Overload the == and && relative to MyClass class.
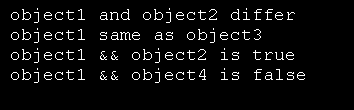
#include <iostream>
using namespace std;
class MyClass {
int x, y;
public:
MyClass() {
x=0;
y=0;
}
MyClass(int i, int j) {
x=i;
y=j;
}
void getXY(int &i, int &j) {
i=x;
j=y;
}
int operator==(MyClass object2);
int operator&&(MyClass object2);
};
// Overload the == operator for MyClass.
int MyClass::operator==(MyClass object2)
{
return x==object2.x && y==object2.y;
}
// Overload the && operator for MyClass.
int MyClass::operator&&(MyClass object2)
{
return (x && object2.x) && (y && object2.y);
}
int main()
{
MyClass object1(10, 10), object2(5, 3), object3(10, 10), object4(0, 0);
if(object1==object2)
cout << "object1 same as object2\n";
else
cout << "object1 and object2 differ\n";
if(object1==object3)
cout << "object1 same as object3\n";
else
cout << "object1 and object3 differ\n";
if(object1 && object2)
cout << "object1 && object2 is true\n";
else
cout << "object1 && object2 is false\n";
if(object1 && object4)
cout << "object1 && object4 is true\n";
else
cout << "object1 && object4 is false\n";
return 0;
}
Related examples in the same category