Demo: Overload ().
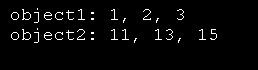
#include <iostream>
using namespace std;
class MyClass {
int x, y, z;
public:
MyClass() {
x = y = z = 0;
}
MyClass(int i, int j, int k) {
x = i;
y = j;
z = k;
}
MyClass operator()(int a, int b, int c);
void show() ;
};
// Overload ().
MyClass MyClass::operator()(int a, int b, int c)
{
MyClass temp;
temp.x = x + a;
temp.y = y + b;
temp.z = z + c;
return temp;
}
void MyClass::show()
{
cout << x << ", ";
cout << y << ", ";
cout << z << endl;
}
int main()
{
MyClass object1(1, 2, 3), object2;
object2 = object1(10, 11, 12); // invoke operator()
cout << "object1: ";
object1.show();
cout << "object2: ";
object2.show();
return 0;
}
Related examples in the same category