Demonstrate overloaded new and delete.
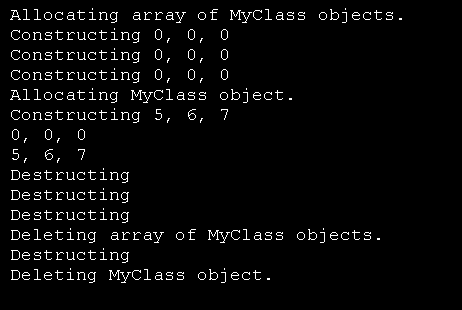
#include <iostream>
#include <new>
#include <cstdlib>
using namespace std;
class MyClass {
int x, y, z;
public:
MyClass() {
x = y = z = 0;
cout << "Constructing 0, 0, 0\n";
}
MyClass(int i, int j, int k) {
x = i;
y = j;
z = k;
cout << "Constructing " << i << ", ";
cout << j << ", " << k;
cout << '\n';
}
~MyClass( ) {
cout << "Destructing\n";
}
void *operator new(size_t size);
void *operator new[](size_t size);
void operator delete(void *p);
void operator delete[](void *p);
void show() ;
};
void *MyClass::operator new(size_t size)
{
void *p;
cout << "Allocating MyClass object.\n";
p = malloc(size);
if(!p) {
bad_alloc ba;
throw ba;
}
return p;
}
void *MyClass::operator new[](size_t size)
{
void *p;
cout << "Allocating array of MyClass objects.\n";
p = malloc(size);
if(!p) {
bad_alloc ba;
throw ba;
}
return p;
}
void MyClass::operator delete(void *p)
{
cout << "Deleting MyClass object.\n";
free(p);
}
void MyClass::operator delete[](void *p)
{
cout << "Deleting array of MyClass objects.\n";
free(p);
}
void MyClass::show()
{
cout << x << ", ";
cout << y << ", ";
cout << z << endl;
}
int main()
{
MyClass *objectPointer1, *objectPointer2;
try {
objectPointer1 = new MyClass[3]; // allocate array
objectPointer2 = new MyClass(5, 6, 7); // allocate object
} catch (bad_alloc ba) {
cout << "Allocation error.\n";
return 1;
}
objectPointer1[1].show();
objectPointer2->show();
delete [] objectPointer1; // delete array
delete objectPointer2; // delete object
return 0;
}
Related examples in the same category