Operator overload: comma operator
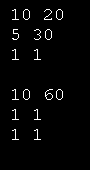
#include <iostream>
using namespace std;
class location {
int longitude, latitude;
public:
location() {}
location(int lg, int lt) {
longitude = lg;
latitude = lt;
}
void show() {
cout << longitude << " ";
cout << latitude << endl;
}
location operator+(location op2);
location operator,(location op2);
};
location location::operator,(location op2)
{
location temp;
temp.longitude = op2.longitude;
temp.latitude = op2.latitude;
cout << op2.longitude << " " << op2.latitude << endl;
return temp;
}
location location::operator+(location op2)
{
location temp;
temp.longitude = op2.longitude + longitude;
temp.latitude = op2.latitude + latitude;
return temp;
}
int main()
{
location object1(10, 20),
object2( 5, 30),
object3(1, 1);
object1.show();
object2.show();
object3.show();
cout << endl;
object1 = (object1, object2+object2, object3);
object1.show();
return 0;
}
Related examples in the same category