Overload the < and >
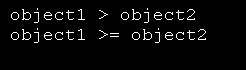
#include <iostream>
using namespace std;
class MyClass {
int x, y;
public:
MyClass() {
x=0;
y=0;
}
MyClass(int i, int j) {
x=i;
y=j;
}
void getXY(int &i, int &j) {
i=x;
j=y;
}
int operator<(MyClass object2);
int operator>(MyClass object2);
};
int MyClass::operator<(MyClass object2)
{
return x<object2.x && y<object2.y;
}
int MyClass::operator>(MyClass object2)
{
return x>object2.x && y>object2.y;
}
int main()
{
MyClass object1(10, 10), object2(5, 3);
if(object1>object2)
cout << "object1 > object2\n";
else
cout << "object1 <= object2 \n";
if(object1<object2)
cout << "object1 < object2\n";
else
cout << "object1 >= object2\n";
return 0;
}
Related examples in the same category