String class: Index characters and '=' operator
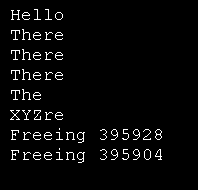
#include <iostream>
#include <cstring>
#include <cstdlib>
using namespace std;
class StringClass {
char *p;
int len;
public:
StringClass(char *s);
~StringClass() {
cout << "Freeing " << (unsigned) p << '\n';
delete [] p;
}
char *get() { return p; }
StringClass &operator=(StringClass &ob);
char &operator[](int i);
};
StringClass::StringClass(char *s)
{
int l;
l = strlen(s)+1;
p = new char [l];
if(!p) {
cout << "Allocation error\n";
exit(1);
}
len = l;
strcpy(p, s);
}
// = operator
StringClass &StringClass::operator=(StringClass &ob)
{
// see if more memory is needed
if(len < ob.len) { // need to allocate more memory
delete [] p;
p = new char [ob.len];
if(!p) {
cout << "Allocation error\n";
exit(1);
}
}
len = ob.len;
strcpy(p, ob.p);
return *this;
}
//
char &StringClass::operator[](int i)
{
if(i <0 || i>len-1) {
cout << "\nIndex value of ";
cout << i << " is out-of-bounds.\n";
exit(1);
}
return p[ i ];
}
int main()
{
StringClass a("Hello"), b("There");
cout << a.get() << '\n';
cout << b.get() << '\n';
a = b; // now p is not overwritten
cout << a.get() << '\n';
cout << b.get() << '\n';
cout << a[0] << a[1] << a[2] << endl;
a[0] = 'X';
a[1] = 'Y';
a[2] = 'Z';
cout << a.get() << endl;
return 0;
}
Related examples in the same category