Example of an icon that changes form
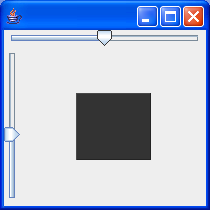
import java.awt.BorderLayout;
import java.awt.Component;
import java.awt.Container;
import java.awt.Graphics;
import javax.swing.Icon;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JSlider;
import javax.swing.event.ChangeEvent;
import javax.swing.event.ChangeListener;
public class DynamicIconExample {
public static void main(String[] args) {
final JSlider width = new JSlider(JSlider.HORIZONTAL, 1, 150, 75);
final JSlider height = new JSlider(JSlider.VERTICAL, 1, 150, 75);
class DynamicIcon implements Icon {
public int getIconWidth() {
return width.getValue();
}
public int getIconHeight() {
return height.getValue();
}
public void paintIcon(Component c, Graphics g, int x, int y) {
g.fill3DRect(x, y, getIconWidth(), getIconHeight(), true);
}
}
Icon icon = new DynamicIcon();
final JLabel dynamicLabel = new JLabel(icon);
class Updater implements ChangeListener {
public void stateChanged(ChangeEvent ev) {
dynamicLabel.repaint();
}
}
Updater updater = new Updater();
width.addChangeListener(updater);
height.addChangeListener(updater);
JFrame f = new JFrame();
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Container c = f.getContentPane();
c.setLayout(new BorderLayout());
c.add(width, BorderLayout.NORTH);
c.add(height, BorderLayout.WEST);
c.add(dynamicLabel, BorderLayout.CENTER);
f.setSize(210, 210);
f.setVisible(true);
}
}
Related examples in the same category