Example showing how to reset the ordering of ImageReaderSpis in Image I/O
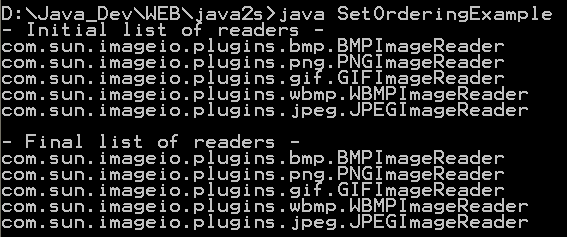
/*
* Copyright (c) 2005 Sun Microsystems, Inc. All Rights Reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* -Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* -Redistribution in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* Neither the name of Sun Microsystems, Inc. or the names of contributors may
* be used to endorse or promote products derived from this software without
* specific prior written permission.
*
* This software is provided "AS IS," without a warranty of any kind. ALL
* EXPRESS OR IMPLIED CONDITIONS, REPRESENTATIONS AND WARRANTIES, INCLUDING ANY
* IMPLIED WARRANTY OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE OR
* NON-INFRINGEMENT, ARE HEREBY EXCLUDED. SUN AND ITS LICENSORS SHALL NOT BE
* LIABLE FOR ANY DAMAGES SUFFERED BY LICENSEE AS A RESULT OF USING, MODIFYING
* OR DISTRIBUTING THE SOFTWARE OR ITS DERIVATIVES. IN NO EVENT WILL SUN OR ITS
* LICENSORS BE LIABLE FOR ANY LOST REVENUE, PROFIT OR DATA, OR FOR DIRECT,
* INDIRECT, SPECIAL, CONSEQUENTIAL, INCIDENTAL OR PUNITIVE DAMAGES, HOWEVER
* CAUSED AND REGARDLESS OF THE THEORY OF LIABILITY, ARISING OUT OF THE USE OF
* OR INABILITY TO USE SOFTWARE, EVEN IF SUN HAS BEEN ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGES.
*
* You acknowledge that Software is not designed,licensed or intended for use in
* the design, construction, operation or maintenance of any nuclear facility.
*/
import java.util.Iterator;
import javax.imageio.spi.IIORegistry;
import javax.imageio.spi.ImageReaderSpi;
import javax.imageio.spi.ServiceRegistry;
/**
* Example showing how to reset the ordering of ImageReaderSpis in Image I/O.
* This is particularly applicable to the case where metadata handling is a
* requirement and is of special importance for the JPEG format as the
* JAI-Image I/O JPEG plug-ins do not handle metadata and have a higher
* priority setting in the IIORegistry than do the core J2SE JPEG plug-ins.
*/
public class SetOrderingExample {
public static void main(String[] args) {
IIORegistry registry = IIORegistry.getDefaultInstance();
// Print the initial ordered list of readers.
System.out.println("- Initial list of readers -");
Iterator providers =
registry.getServiceProviders(ImageReaderSpi.class, true);
while(providers.hasNext()) {
ImageReaderSpi provider = (ImageReaderSpi) providers.next();
System.out.println(provider.getPluginClassName());
}
System.out.println("");
// Get the reader SPIs for JPEG.
providers = registry.getServiceProviders(ImageReaderSpi.class,
new JPEGFilter(),
true);
// Save reader SPIs for JPEG according to whether they support
// native image metadata. This removes the dependence on knowing
// the class name of the associated reader plug-in.
ImageReaderSpi readerMetadataSPI = null;
ImageReaderSpi readerNoMetadataSPI = null;
while(providers.hasNext()) {
ImageReaderSpi readerSPI = (ImageReaderSpi)providers.next();
if(readerSPI.getNativeImageMetadataFormatName() != null) {
readerMetadataSPI = readerSPI;
} else {
readerNoMetadataSPI = readerSPI;
}
}
// Update ordering if both metadata-capable and -incapable readers
// are detected.
if(readerMetadataSPI != null && readerNoMetadataSPI != null) {
// Remove ordering that places metadata-capable reader last.
boolean unsetResult = registry.unsetOrdering(ImageReaderSpi.class,
readerNoMetadataSPI,
readerMetadataSPI);
// Add ordering that places metadata-capable reader first.
boolean setResult = registry.setOrdering(ImageReaderSpi.class,
readerMetadataSPI,
readerNoMetadataSPI);
}
// Print the final ordered list of readers.
System.out.println("- Final list of readers -");
providers = registry.getServiceProviders(ImageReaderSpi.class, true);
while(providers.hasNext()) {
ImageReaderSpi provider = (ImageReaderSpi) providers.next();
System.out.println(provider.getPluginClassName());
}
}
}
/**
* Filter which returns <code>true</code> if and only if the provider is
* an <code>ImageReaderSpi</code> which supports the JPEG format.
*/
class JPEGFilter implements ServiceRegistry.Filter {
JPEGFilter() {}
public boolean filter(Object provider) {
if(!(provider instanceof ImageReaderSpi)) {
return false;
}
ImageReaderSpi readerSPI = (ImageReaderSpi)provider;
String[] formatNames = readerSPI.getFormatNames();
for(int i = 0; i < formatNames.length; i++) {
if(formatNames[i].equalsIgnoreCase("JPEG")) {
return true;
}
}
return false;
}
}
Related examples in the same category