Simple demo of avoiding side-effects by using Object.clone
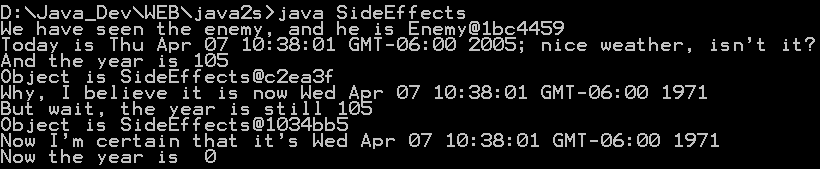
/*
* Copyright (c) Ian F. Darwin, http://www.darwinsys.com/, 1996-2002.
* All rights reserved. Software written by Ian F. Darwin and others.
* $Id: LICENSE,v 1.8 2004/02/09 03:33:38 ian Exp $
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY THE AUTHOR AND CONTRIBUTORS ``AS IS''
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED
* TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE AUTHOR OR CONTRIBUTORS
* BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* Java, the Duke mascot, and all variants of Sun's Java "steaming coffee
* cup" logo are trademarks of Sun Microsystems. Sun's, and James Gosling's,
* pioneering role in inventing and promulgating (and standardizing) the Java
* language and environment is gratefully acknowledged.
*
* The pioneering role of Dennis Ritchie and Bjarne Stroustrup, of AT&T, for
* inventing predecessor languages C and C++ is also gratefully acknowledged.
*/
import java.util.*;
/**
* Simple demo of avoiding side-effects by using Object.clone()
* to duplicate an object before passing it to your enemy's methods.
* Cloneable is a "marker" interface: it has no methods, but is tested
* for by Object.clone. If you implement it, you tell Object.clone that
* your data is stable enough that field-by-field copy is OK.
*/
class Enemy {
public void munge(SideEffects md) {
System.out.println("Object is " + md);
md.year = 0;
md.td.setYear(71); // Ignore deprecation warnings
}
}
public class SideEffects implements Cloneable {
/** When we clone a "SideEffects", this REFERENCE gets cloned */
public Date td;
/** When we clone a "SideEffects", this integer does NOT get cloned */
volatile int year;
public static void main(String[] argv) throws CloneNotSupportedException {
new SideEffects().process();
}
SideEffects() {
td = new Date(); // today
year = td.getYear();
}
public void process() throws CloneNotSupportedException {
Enemy r = new Enemy();
System.out.println("We have seen the enemy, and he is " + r);
System.out.println("Today is " + td + "; nice weather, isn't it?");
System.out.println("And the year is " + year);
r.munge((SideEffects)this.clone());
System.out.println("Why, I believe it is now " + td);
if (year == 0) // should not happen!!
System.out.println("** PANIC IN YEAR ZERO **");
System.out.println("But wait, the year is still " + year);
r.munge(this);
System.out.println("Now I'm certain that it's " + td);
System.out.println("Now the year is " + year);
}
}
Related examples in the same category