Converts a Array to an Enumeration and allows it to be serialized
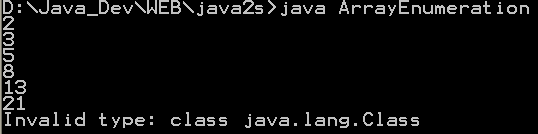
/*
* Copyright Javelin Software, All rights reserved.
*/
import java.util.*;
import java.io.*;
/**
* An ArrayEnumeration converts a Array to an Enumeration and allows it
* to be serialized.
* <p>
* @author Robin Sharp
*/
public class ArrayEnumeration implements Enumeration, Serializable
{
/**
* Construct a fully qualified ArrayEnumeration.
* @param array the array to be Enumerated.
*/
public ArrayEnumeration(Object[] array)
{
this.array = array;
}
// ENUMERATION /////////////////////////////////////////////////////////
/**
* @return true if there are more elements in the enumeration.
*/
public boolean hasMoreElements()
{
return array != null && index < array.length;
}
/**
* @return the next element in the enumeration
*/
public Object nextElement()
{
return array[index++];
}
// PRIVATE /////////////////////////////////////////////////////////////
private Object[] array;
private int index;
}
Related examples in the same category