Text Tab Sample
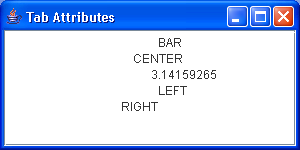
/*
Definitive Guide to Swing for Java 2, Second Edition
By John Zukowski
ISBN: 1-893115-78-X
Publisher: APress
*/
import java.awt.BorderLayout;
import java.awt.Container;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTextPane;
import javax.swing.text.BadLocationException;
import javax.swing.text.DefaultStyledDocument;
import javax.swing.text.SimpleAttributeSet;
import javax.swing.text.StyleConstants;
import javax.swing.text.StyledDocument;
import javax.swing.text.TabSet;
import javax.swing.text.TabStop;
public class TextTabSample {
public static void main(String args[]) {
JFrame frame = new JFrame("Tab Attributes");
Container content = frame.getContentPane();
StyledDocument document = new DefaultStyledDocument();
int positions[] = { TabStop.ALIGN_BAR, TabStop.ALIGN_CENTER,
TabStop.ALIGN_DECIMAL, TabStop.ALIGN_LEFT, TabStop.ALIGN_RIGHT };
String strings[] = { "\tBAR\n", "\tCENTER\n", "\t3.14159265\n",
"\tLEFT\n", "\tRIGHT\n" };
SimpleAttributeSet attributes = new SimpleAttributeSet();
for (int i = 0, n = positions.length; i < n; i++) {
TabStop tabstop = new TabStop(150, positions[i], TabStop.LEAD_DOTS);
try {
int position = document.getLength();
document.insertString(position, strings[i], null);
TabSet tabset = new TabSet(new TabStop[] { tabstop });
StyleConstants.setTabSet(attributes, tabset);
document.setParagraphAttributes(position, 1, attributes, false);
} catch (BadLocationException badLocationException) {
System.err.println("Oops");
}
}
JTextPane textPane = new JTextPane(document);
textPane.setEditable(false);
JScrollPane scrollPane = new JScrollPane(textPane);
content.add(scrollPane, BorderLayout.CENTER);
frame.setSize(300, 150);
frame.setVisible(true);
}
}
Related examples in the same category