Showing how to add Actions for KeyStrokes
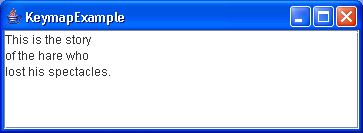
import java.awt.BorderLayout;
import java.awt.event.ActionEvent;
import java.awt.event.InputEvent;
import java.awt.event.KeyEvent;
import java.util.Hashtable;
import javax.swing.Action;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.KeyStroke;
import javax.swing.text.BadLocationException;
import javax.swing.text.DefaultEditorKit;
import javax.swing.text.Document;
import javax.swing.text.JTextComponent;
import javax.swing.text.Keymap;
import javax.swing.text.TextAction;
public class KeymapExample {
public static void main(String[] args) {
JTextArea area = new JTextArea(6, 32);
Keymap parent = area.getKeymap();
Keymap newmap = JTextComponent.addKeymap("KeymapExampleMap", parent);
KeyStroke u = KeyStroke.getKeyStroke(KeyEvent.VK_U,
InputEvent.CTRL_MASK);
Action actionU = new UpWord();
newmap.addActionForKeyStroke(u, actionU);
Action actionList[] = area.getActions();
Hashtable lookup = new Hashtable();
for (int j = 0; j < actionList.length; j += 1)
lookup.put(actionList[j].getValue(Action.NAME), actionList[j]);
KeyStroke L = KeyStroke.getKeyStroke(KeyEvent.VK_L,
InputEvent.CTRL_MASK);
Action actionL = (Action) lookup.get(DefaultEditorKit.selectLineAction);
newmap.addActionForKeyStroke(L, actionL);
KeyStroke W = KeyStroke.getKeyStroke(KeyEvent.VK_W,
InputEvent.CTRL_MASK);
Action actionW = (Action) lookup.get(DefaultEditorKit.selectWordAction);
newmap.addActionForKeyStroke(W, actionW);
area.setKeymap(newmap);
JFrame f = new JFrame("KeymapExample");
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.getContentPane().add(new JScrollPane(area), BorderLayout.CENTER);
area.setText("This is a test.");
f.pack();
f.setVisible(true);
}
}
class UpWord extends TextAction {
public UpWord() {
super("uppercase-word-action");
}
public void actionPerformed(ActionEvent e) {
JTextComponent comp = getTextComponent(e);
if (comp == null)
return;
Document doc = comp.getDocument();
int start = comp.getSelectionStart();
int end = comp.getSelectionEnd();
try {
int left = javax.swing.text.Utilities.getWordStart(comp, start);
int right = javax.swing.text.Utilities.getWordEnd(comp, end);
String word = doc.getText(left, right - left);
doc.remove(left, right - left);
doc.insertString(left, word.toUpperCase(), null);
comp.setSelectionStart(start);
comp.setSelectionEnd(end);
} catch (BadLocationException ble) {
return;
}
}
}
Related examples in the same category