Grid Data Bound Drag and Drop Sample (Smart GWT)
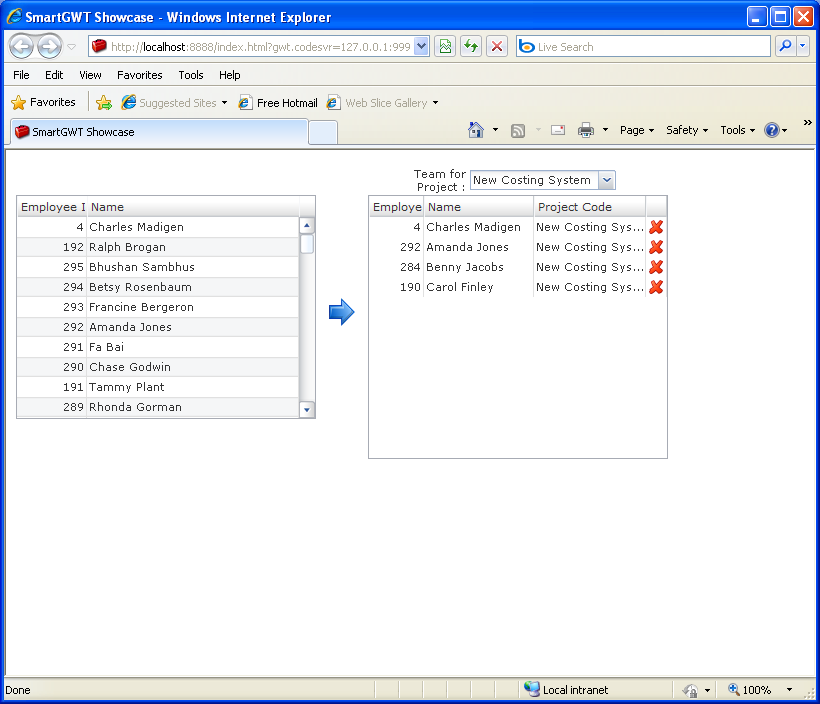
/*
* SmartGWT (GWT for SmartClient)
* Copyright 2008 and beyond, Isomorphic Software, Inc.
*
* SmartGWT is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License version 3
* as published by the Free Software Foundation. SmartGWT is also
* available under typical commercial license terms - see
* http://smartclient.com/license
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*/
package com.smartgwt.sample.showcase.client;
import java.util.Date;
import com.google.gwt.core.client.EntryPoint;
import com.google.gwt.user.client.ui.RootPanel;
import com.smartgwt.client.data.Criteria;
import com.smartgwt.client.data.DSRequest;
import com.smartgwt.client.data.DataSource;
import com.smartgwt.client.data.fields.DataSourceBooleanField;
import com.smartgwt.client.data.fields.DataSourceDateField;
import com.smartgwt.client.data.fields.DataSourceEnumField;
import com.smartgwt.client.data.fields.DataSourceFloatField;
import com.smartgwt.client.data.fields.DataSourceIntegerField;
import com.smartgwt.client.data.fields.DataSourceTextField;
import com.smartgwt.client.types.Alignment;
import com.smartgwt.client.types.DragDataAction;
import com.smartgwt.client.widgets.Canvas;
import com.smartgwt.client.widgets.Img;
import com.smartgwt.client.widgets.events.ClickEvent;
import com.smartgwt.client.widgets.events.ClickHandler;
import com.smartgwt.client.widgets.form.DynamicForm;
import com.smartgwt.client.widgets.form.fields.SelectItem;
import com.smartgwt.client.widgets.form.fields.events.ChangedEvent;
import com.smartgwt.client.widgets.form.fields.events.ChangedHandler;
import com.smartgwt.client.widgets.form.validator.FloatPrecisionValidator;
import com.smartgwt.client.widgets.form.validator.FloatRangeValidator;
import com.smartgwt.client.widgets.grid.ListGrid;
import com.smartgwt.client.widgets.grid.ListGridField;
import com.smartgwt.client.widgets.grid.ListGridRecord;
import com.smartgwt.client.widgets.layout.HStack;
import com.smartgwt.client.widgets.layout.LayoutSpacer;
import com.smartgwt.client.widgets.layout.VStack;
public class Showcase implements EntryPoint {
public void onModuleLoad() {
RootPanel.get().add(getViewPanel());
}
public Canvas getViewPanel() {
String[] projects = new String[] { "New Costing System", "Warehousing Improvements",
"Evaluate AJAX Frameworks", "Upgrade Postgres", "Online Billing" };
DataSource employeesDS = EmployeeXmlDS.getInstance();
final ListGrid employeesGrid = new ListGrid();
employeesGrid.setWidth(300);
employeesGrid.setHeight(224);
employeesGrid.setDataSource(employeesDS);
employeesGrid.setCanDragRecordsOut(true);
employeesGrid.setDragDataAction(DragDataAction.COPY);
employeesGrid.setAlternateRecordStyles(true);
employeesGrid.setAutoFetchData(false);
ListGridField employeeIdField = new ListGridField("EmployeeId");
employeeIdField.setWidth("25%");
ListGridField nameField = new ListGridField("Name");
employeesGrid.setFields(employeeIdField, nameField);
DataSource teamMembersDS = TeamMembersXmlDS.getInstance();
final ListGrid projectGrid = new ListGrid();
projectGrid.setWidth(300);
projectGrid.setHeight(264);
projectGrid.setDataSource(teamMembersDS);
projectGrid.setCanAcceptDroppedRecords(true);
projectGrid.setCanRemoveRecords(true);
projectGrid.setAutoFetchData(false);
projectGrid.setPreventDuplicates(true);
ListGridField employeeIdField2 = new ListGridField("employeeId");
employeeIdField2.setWidth("20%");
ListGridField employeeNameField2 = new ListGridField("employeeName");
employeeNameField2.setWidth("40%");
ListGridField projectCodeField2 = new ListGridField("projectCode");
projectGrid.setFields(employeeIdField2, employeeNameField2, projectCodeField2);
HStack hStack = new HStack(10);
hStack.setHeight(160);
VStack vStack = new VStack();
LayoutSpacer spacer = new LayoutSpacer();
spacer.setHeight(30);
vStack.addMember(spacer);
vStack.addMember(employeesGrid);
hStack.addMember(vStack);
Img arrowImg = new Img("icons/32/arrow_right.png", 32, 32);
arrowImg.setLayoutAlign(Alignment.CENTER);
arrowImg.addClickHandler(new ClickHandler() {
public void onClick(ClickEvent event) {
projectGrid.transferSelectedData(employeesGrid);
}
});
hStack.addMember(arrowImg);
VStack vStack2 = new VStack();
final DynamicForm projectSelectorForm = new DynamicForm();
projectSelectorForm.setWidth(300);
projectSelectorForm.setHeight(30);
SelectItem selectItem = new SelectItem("projectCode", "Team for Project");
selectItem.setDefaultValue(projects[0]);
selectItem.setValueMap(projects);
selectItem.addChangedHandler(new ChangedHandler() {
public void onChanged(ChangedEvent event) {
Criteria criteria = projectSelectorForm.getValuesAsCriteria();
projectGrid.fetchData(criteria);
}
});
projectSelectorForm.setFields(selectItem);
vStack2.addMember(projectSelectorForm);
vStack2.addMember(projectGrid);
hStack.addMember(vStack2);
projectGrid.fetchData(projectSelectorForm.getValuesAsCriteria());
employeesGrid.fetchData();
return hStack;
}
}
class TeamMembersXmlDS extends DataSource {
private static TeamMembersXmlDS instance = null;
public static TeamMembersXmlDS getInstance() {
if (instance == null) {
instance = new TeamMembersXmlDS("teamMembersDS");
}
return instance;
}
public TeamMembersXmlDS(String id) {
setID(id);
setTitleField("employeeName");
setRecordXPath("/List/teamMember");
DataSourceIntegerField syntheticField = new DataSourceIntegerField("uniqueSeq");
syntheticField.setHidden(true);
syntheticField.setPrimaryKey(true);
DataSourceTextField nameField = new DataSourceTextField("employeeName", "Name", 128);
DataSourceIntegerField employeeIdField = new DataSourceIntegerField("employeeId", "Employee ID");
employeeIdField.setForeignKey("employeesDS.EmployeeId");
DataSourceTextField projectCodeField = new DataSourceTextField("projectCode", "Project Code", 30);
setFields(syntheticField, nameField, employeeIdField, projectCodeField);
setDataURL("ds/test_data/teamMembers.data.xml");
setClientOnly(true);
}
}
class EmployeeXmlDS extends DataSource {
private static EmployeeXmlDS instance = null;
public static EmployeeXmlDS getInstance() {
if (instance == null) {
instance = new EmployeeXmlDS("employeesDS");
}
return instance;
}
public EmployeeXmlDS(String id) {
setID(id);
setTitleField("Name");
setRecordXPath("/List/employee");
DataSourceTextField nameField = new DataSourceTextField("Name", "Name", 128);
DataSourceIntegerField employeeIdField = new DataSourceIntegerField("EmployeeId", "Employee ID");
employeeIdField.setPrimaryKey(true);
employeeIdField.setRequired(true);
DataSourceIntegerField reportsToField = new DataSourceIntegerField("ReportsTo", "Manager");
reportsToField.setRequired(true);
reportsToField.setForeignKey(id + ".EmployeeId");
reportsToField.setRootValue("1");
DataSourceTextField jobField = new DataSourceTextField("Job", "Title", 128);
DataSourceTextField emailField = new DataSourceTextField("Email", "Email", 128);
DataSourceTextField statusField = new DataSourceTextField("EmployeeStatus", "Status", 40);
DataSourceFloatField salaryField = new DataSourceFloatField("Salary", "Salary");
DataSourceTextField orgField = new DataSourceTextField("OrgUnit", "Org Unit", 128);
DataSourceTextField genderField = new DataSourceTextField("Gender", "Gender", 7);
genderField.setValueMap("male", "female");
DataSourceTextField maritalStatusField = new DataSourceTextField("MaritalStatus", "Marital Status", 10);
setFields(nameField, employeeIdField, reportsToField, jobField, emailField,
statusField, salaryField, orgField, genderField, maritalStatusField);
setDataURL("ds/test_data/employees.data.xml");
setClientOnly(true);
}
}
SmartGWT.zip( 9,880 k)Related examples in the same category