Grid Form Add Sample (Smart GWT)
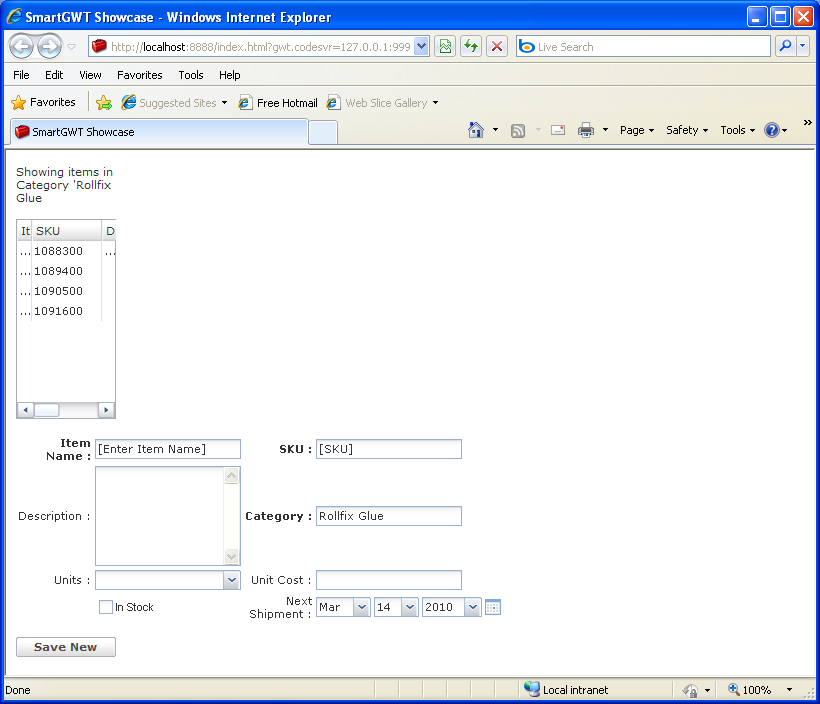
/*
* SmartGWT (GWT for SmartClient)
* Copyright 2008 and beyond, Isomorphic Software, Inc.
*
* SmartGWT is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License version 3
* as published by the Free Software Foundation. SmartGWT is also
* available under typical commercial license terms - see
* http://smartclient.com/license
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*/
package com.smartgwt.sample.showcase.client;
import java.util.Date;
import com.google.gwt.core.client.EntryPoint;
import com.google.gwt.user.client.ui.RootPanel;
import com.smartgwt.client.data.DSRequest;
import com.smartgwt.client.data.DataSource;
import com.smartgwt.client.data.fields.DataSourceBooleanField;
import com.smartgwt.client.data.fields.DataSourceDateField;
import com.smartgwt.client.data.fields.DataSourceEnumField;
import com.smartgwt.client.data.fields.DataSourceFloatField;
import com.smartgwt.client.data.fields.DataSourceIntegerField;
import com.smartgwt.client.data.fields.DataSourceSequenceField;
import com.smartgwt.client.data.fields.DataSourceTextField;
import com.smartgwt.client.widgets.Canvas;
import com.smartgwt.client.widgets.IButton;
import com.smartgwt.client.widgets.Label;
import com.smartgwt.client.widgets.events.ClickEvent;
import com.smartgwt.client.widgets.events.ClickHandler;
import com.smartgwt.client.widgets.form.DynamicForm;
import com.smartgwt.client.widgets.form.validator.FloatPrecisionValidator;
import com.smartgwt.client.widgets.form.validator.FloatRangeValidator;
import com.smartgwt.client.widgets.grid.ListGrid;
import com.smartgwt.client.widgets.grid.ListGridRecord;
import com.smartgwt.client.widgets.layout.VLayout;
public class Showcase implements EntryPoint {
public void onModuleLoad() {
RootPanel.get().add(getViewPanel());
}
public Canvas getViewPanel() {
VLayout layout = new VLayout(15);
Label label = new Label();
label.setHeight(10);
label.setWidth100();
label.setContents("Showing items in Category 'Rollfix Glue");
layout.addMember(label);
final DataSource dataSource = ItemSupplyLocalDS.getInstance();
ListGrid listGrid = new ListGrid();
listGrid.setWidth100();
listGrid.setHeight(200);
listGrid.setDataSource(dataSource);
listGrid.setAutoFetchData(true);
layout.addMember(listGrid);
final DynamicForm form = new DynamicForm();
form.setNumCols(4);
form.setDataSource(dataSource);
form.setValue("category", "Rollfix Glue");
form.setValue("itemName", "[Enter Item Name]");
form.setValue("SKU", "[SKU]");
form.setValue("unitCoset", "[Enter Price]");
layout.addMember(form);
IButton button = new IButton("Save New");
button.addClickHandler(new ClickHandler() {
public void onClick(ClickEvent event) {
form.saveData();
form.reset();
}
});
layout.addMember(button);
return layout;
}
}
class ItemSupplyLocalDS extends DataSource {
private static ItemSupplyLocalDS instance = null;
public static ItemSupplyLocalDS getInstance() {
if (instance == null) {
instance = new ItemSupplyLocalDS("supplyItemLocalDS");
}
return instance;
}
public ItemSupplyLocalDS(String id) {
setID(id);
DataSourceIntegerField pkField = new DataSourceIntegerField("itemID");
pkField.setHidden(true);
pkField.setPrimaryKey(true);
DataSourceTextField itemNameField = new DataSourceTextField("itemName", "Item Name", 128, true);
DataSourceTextField skuField = new DataSourceTextField("SKU", "SKU", 10, true);
DataSourceTextField descriptionField = new DataSourceTextField("description", "Description",
2000);
DataSourceTextField categoryField = new DataSourceTextField("category", "Category", 128, true);
categoryField.setForeignKey("supplyCategory.categoryName");
DataSourceEnumField unitsField = new DataSourceEnumField("units", "Units", 5);
unitsField.setValueMap("Roll", "Ea", "Pkt", "Set", "Tube", "Pad", "Ream", "Tin", "Bag", "Ctn",
"Box");
DataSourceFloatField unitCostField = new DataSourceFloatField("unitCost", "Unit Cost", 5);
FloatRangeValidator rangeValidator = new FloatRangeValidator();
rangeValidator.setMin(0);
rangeValidator.setErrorMessage("Please enter a valid (positive) cost");
FloatPrecisionValidator precisionValidator = new FloatPrecisionValidator();
precisionValidator.setPrecision(2);
precisionValidator.setErrorMessage("The maximum allowed precision is 2");
unitCostField.setValidators(rangeValidator, precisionValidator);
DataSourceBooleanField inStockField = new DataSourceBooleanField("inStock", "In Stock");
DataSourceDateField nextShipmentField = new DataSourceDateField("nextShipment", "Next Shipment");
setFields(pkField, itemNameField, skuField, descriptionField, categoryField, unitsField,
unitCostField, inStockField, nextShipmentField);
setClientOnly(true);
setTestData(ItemData.getRecords());
}
protected Object transformRequest(DSRequest dsRequest) {
return super.transformRequest(dsRequest);
}
}
class ItemData {
private static ItemRecord[] records;
public static ItemRecord[] getRecords() {
if (records == null) {
records = getNewRecords();
}
return records;
}
public static ItemRecord[] getNewRecords() {
return new ItemRecord[]{
new ItemRecord(1, "Glue Pelikan Roll-fix Permanent #950", "1088300", "A clean no-drips, no brush roll-on glue. Will not dry out. Suitable for artwork, photos or posters. Glue cartridges are 12 metres long. Refillable.", "Rollfix Glue", "Ea", 6.96, null, null),
new ItemRecord(2, "Glue Pelikan Roll-fix Refill Permanent #955", "1089400", null, "Rollfix Glue", "Ea", 3.73, null, null),
new ItemRecord(3, "Glue Pelikan Roll-fix Non Permanent #960", "1090500", null, "Rollfix Glue", "Ea", 6.96, null, null),
new ItemRecord(4, "Glue Pelikan Roll-fix Refill Non Permanent #965", "1091600", null, "Rollfix Glue", "Ea", 3.73, null, null)
};
}
}
class ItemRecord extends ListGridRecord {
public ItemRecord() {
}
public ItemRecord(int itemID, String item, String sku, String description, String category, String units, Double unitCost, Boolean inStock, Date nextShipment) {
setItemID(itemID);
setItemName(item);
setSKU(sku);
setDescription(description);
setCategory(category);
setUnits(units);
setUnitCost(unitCost);
setInStock(inStock);
setNextShipment(nextShipment);
}
/**
* Set the itemID.
*
* @param itemID the itemID
*/
public void setItemID(int itemID) {
setAttribute("itemID", itemID);
}
/**
* Return the itemID.
*
* @return the itemID
*/
public int getItemID() {
return getAttributeAsInt("itemID");
}
/**
* Set the item.
*
* @param item the item
*/
public void setItemName(String item) {
setAttribute("itemName", item);
}
/**
* Return the item.
*
* @return the item
*/
public String getItemName() {
return getAttribute("itemName");
}
/**
* Set the SKU.
*
* @param SKU the SKU
*/
public void setSKU(String SKU) {
setAttribute("SKU", SKU);
}
/**
* Return the SKU.
*
* @return the SKU
*/
public String getSKU() {
return getAttribute("SKU");
}
/**
* Set the description.
*
* @param description the description
*/
public void setDescription(String description) {
setAttribute("description", description);
}
/**
* Return the description.
*
* @return the description
*/
public String getDescription() {
return getAttribute("description");
}
/**
* Set the category.
*
* @param category the category
*/
public void setCategory(String category) {
setAttribute("category", category);
}
/**
* Return the category.
*
* @return the category
*/
public String getCategory() {
return getAttribute("category");
}
/**
* Set the units.
*
* @param units the units
*/
public void setUnits(String units) {
setAttribute("units", units);
}
/**
* Return the units.
*
* @return the units
*/
public String getUnits() {
return getAttribute("units");
}
/**
* Set the unitCost.
*
* @param unitCost the unitCost
*/
public void setUnitCost(Double unitCost) {
setAttribute("unitCost", unitCost);
}
/**
* Return the unitCost.
*
* @return the unitCost
*/
public Float getUnitCost() {
return getAttributeAsFloat("unitCost");
}
/**
* Set the inStock.
*
* @param inStock the inStock
*/
public void setInStock(Boolean inStock) {
setAttribute("inStock", inStock);
}
/**
* Return the inStock.
*
* @return the inStock
*/
public Boolean getInStock() {
return getAttributeAsBoolean("inStock");
}
/**
* Set the nextShipment.
*
* @param nextShipment the nextShipment
*/
public void setNextShipment(Date nextShipment) {
setAttribute("nextShipment", nextShipment);
}
/**
* Return the nextShipment.
*
* @return the nextShipment
*/
public Date getNextShipment() {
return getAttributeAsDate("nextShipment");
}
}
SmartGWT.zip( 9,880 k)Related examples in the same category