Grid and Form driven by the DataSource definition and no code to displaying labels or fields (Smart GWT)
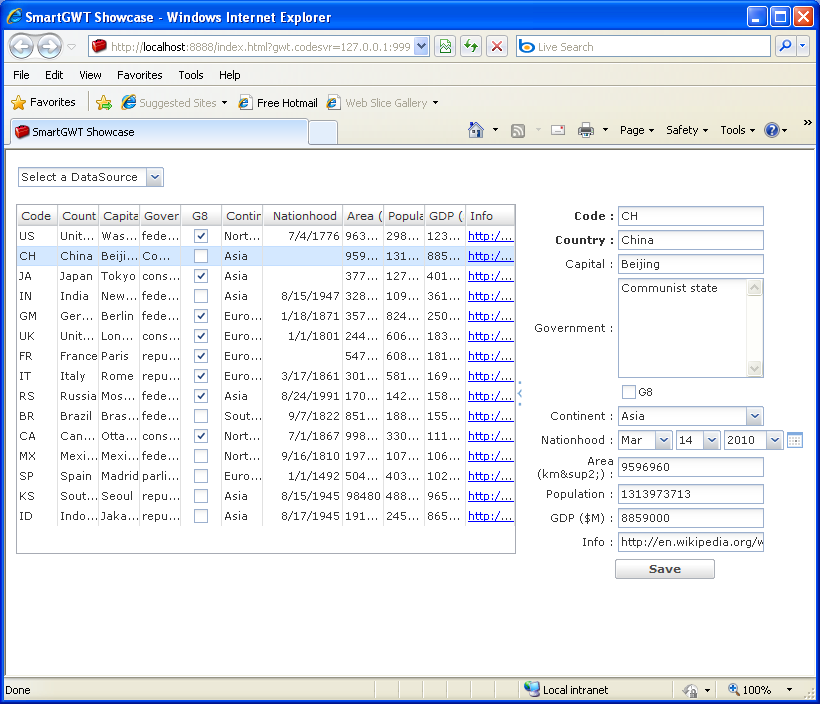
/*
* SmartGWT (GWT for SmartClient)
* Copyright 2008 and beyond, Isomorphic Software, Inc.
*
* SmartGWT is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License version 3
* as published by the Free Software Foundation. SmartGWT is also
* available under typical commercial license terms - see
* http://smartclient.com/license
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*/
package com.smartgwt.sample.showcase.client;
import com.google.gwt.core.client.EntryPoint;
import com.google.gwt.user.client.ui.RootPanel;
import com.smartgwt.client.data.DataSource;
import com.smartgwt.client.data.fields.DataSourceBooleanField;
import com.smartgwt.client.data.fields.DataSourceDateField;
import com.smartgwt.client.data.fields.DataSourceEnumField;
import com.smartgwt.client.data.fields.DataSourceFloatField;
import com.smartgwt.client.data.fields.DataSourceIntegerField;
import com.smartgwt.client.data.fields.DataSourceLinkField;
import com.smartgwt.client.data.fields.DataSourceTextField;
import com.smartgwt.client.types.Alignment;
import com.smartgwt.client.widgets.Canvas;
import com.smartgwt.client.widgets.IButton;
import com.smartgwt.client.widgets.events.ClickEvent;
import com.smartgwt.client.widgets.events.ClickHandler;
import com.smartgwt.client.widgets.form.DynamicForm;
import com.smartgwt.client.widgets.form.fields.SelectItem;
import com.smartgwt.client.widgets.form.fields.events.ChangedEvent;
import com.smartgwt.client.widgets.form.fields.events.ChangedHandler;
import com.smartgwt.client.widgets.form.validator.FloatPrecisionValidator;
import com.smartgwt.client.widgets.form.validator.FloatRangeValidator;
import com.smartgwt.client.widgets.grid.ListGrid;
import com.smartgwt.client.widgets.grid.events.RecordClickEvent;
import com.smartgwt.client.widgets.grid.events.RecordClickHandler;
import com.smartgwt.client.widgets.layout.HLayout;
import com.smartgwt.client.widgets.layout.VLayout;
public class Showcase implements EntryPoint {
public void onModuleLoad() {
RootPanel.get().add(getViewPanel());
}
public Canvas getViewPanel() {
final DataSource countryDS = CountryXmlDS.getInstance();
final DataSource supplyItemDS = ItemSupplyXmlDS.getInstance();
final CompoundEditor cEditor = new CompoundEditor(countryDS);
SelectItem dsSelect = new SelectItem();
dsSelect.setName("datasource");
dsSelect.setShowTitle(false);
dsSelect.setValueMap("country", "supply");
dsSelect.addChangedHandler(new ChangedHandler() {
public void onChanged(ChangedEvent event) {
String ds = (String) event.getValue();
if (ds.equalsIgnoreCase("country")) {
cEditor.setDatasource(countryDS);
} else {
cEditor.setDatasource(supplyItemDS);
}
}
});
DynamicForm form = new DynamicForm();
form.setValue("datasource", "Select a DataSource");
form.setItems(dsSelect);
VLayout layout = new VLayout(15);
layout.setWidth100();
layout.setHeight("80%");
layout.addMember(form);
layout.addMember(cEditor);
return layout;
}
private static class CompoundEditor extends HLayout {
private DataSource datasource;
private DynamicForm form;
private ListGrid grid;
private IButton saveButton;
private CompoundEditor(DataSource datasource) {
this.datasource = datasource;
}
protected void onInit() {
super.onInit();
this.form = new DynamicForm();
form.setDataSource(datasource);
saveButton = new IButton("Save");
saveButton.setLayoutAlign(Alignment.CENTER);
saveButton.addClickHandler(new ClickHandler() {
public void onClick(ClickEvent event) {
form.saveData();
}
});
VLayout editorLayout = new VLayout(5);
editorLayout.addMember(form);
editorLayout.addMember(saveButton);
editorLayout.setWidth(280);
grid = new ListGrid();
grid.setWidth(500);
grid.setHeight(350);
grid.setDataSource(datasource);
grid.setShowResizeBar(true);
grid.setAutoFetchData(true);
grid.addRecordClickHandler(new RecordClickHandler() {
public void onRecordClick(RecordClickEvent event) {
form.clearErrors(true);
form.editRecord(event.getRecord());
saveButton.enable();
}
});
addMember(grid);
addMember(editorLayout);
}
public DataSource getDatasource() {
return datasource;
}
public void setDatasource(DataSource datasource) {
this.datasource = datasource;
grid.setDataSource(datasource);
form.setDataSource(datasource);
saveButton.disable();
grid.fetchData();
}
}
}
class ItemSupplyXmlDS extends DataSource {
private static ItemSupplyXmlDS instance = null;
public static ItemSupplyXmlDS getInstance() {
if (instance == null) {
instance = new ItemSupplyXmlDS("supplyItemDS");
}
return instance;
}
public ItemSupplyXmlDS(String id) {
setID(id);
setRecordXPath("/List/supplyItem");
DataSourceIntegerField pkField = new DataSourceIntegerField("itemID");
pkField.setHidden(true);
pkField.setPrimaryKey(true);
DataSourceTextField itemNameField = new DataSourceTextField("itemName", "Item Name", 128, true);
DataSourceTextField skuField = new DataSourceTextField("SKU", "SKU", 10, true);
DataSourceTextField descriptionField = new DataSourceTextField("description", "Description",
2000);
DataSourceTextField categoryField = new DataSourceTextField("category", "Category", 128, true);
categoryField.setForeignKey("supplyCategoryDS.categoryName");
DataSourceEnumField unitsField = new DataSourceEnumField("units", "Units", 5);
unitsField.setValueMap("Roll", "Ea", "Pkt", "Set", "Tube", "Pad", "Ream", "Tin", "Bag", "Ctn",
"Box");
DataSourceFloatField unitCostField = new DataSourceFloatField("unitCost", "Unit Cost", 5);
FloatRangeValidator rangeValidator = new FloatRangeValidator();
rangeValidator.setMin(0);
rangeValidator.setErrorMessage("Please enter a valid (positive) cost");
FloatPrecisionValidator precisionValidator = new FloatPrecisionValidator();
precisionValidator.setPrecision(2);
precisionValidator.setErrorMessage("The maximum allowed precision is 2");
unitCostField.setValidators(rangeValidator, precisionValidator);
DataSourceBooleanField inStockField = new DataSourceBooleanField("inStock", "In Stock");
DataSourceDateField nextShipmentField = new DataSourceDateField("nextShipment", "Next Shipment");
setFields(pkField, itemNameField, skuField, descriptionField, categoryField, unitsField,
unitCostField, inStockField, nextShipmentField);
setDataURL("ds/test_data/supplyItem.data.xml");
setClientOnly(true);
}
}
class CountryXmlDS extends DataSource {
private static CountryXmlDS instance = null;
public static CountryXmlDS getInstance() {
if (instance == null) {
instance = new CountryXmlDS("countryDS");
}
return instance;
}
public CountryXmlDS(String id) {
setID(id);
setRecordXPath("/List/country");
DataSourceIntegerField pkField = new DataSourceIntegerField("pk");
pkField.setHidden(true);
pkField.setPrimaryKey(true);
DataSourceTextField countryCodeField = new DataSourceTextField("countryCode", "Code");
countryCodeField.setRequired(true);
DataSourceTextField countryNameField = new DataSourceTextField("countryName", "Country");
countryNameField.setRequired(true);
DataSourceTextField capitalField = new DataSourceTextField("capital", "Capital");
DataSourceTextField governmentField = new DataSourceTextField("government", "Government", 500);
DataSourceBooleanField memberG8Field = new DataSourceBooleanField("member_g8", "G8");
DataSourceTextField continentField = new DataSourceTextField("continent", "Continent");
continentField.setValueMap("Europe", "Asia", "North America", "Australia/Oceania",
"South America", "Africa");
DataSourceDateField independenceField = new DataSourceDateField("independence", "Nationhood");
DataSourceFloatField areaField = new DataSourceFloatField("area", "Area (km²)");
DataSourceIntegerField populationField = new DataSourceIntegerField("population", "Population");
DataSourceFloatField gdpField = new DataSourceFloatField("gdp", "GDP ($M)");
DataSourceLinkField articleField = new DataSourceLinkField("article", "Info");
setFields(pkField, countryCodeField, countryNameField, capitalField, governmentField,
memberG8Field, continentField, independenceField, areaField, populationField, gdpField,
articleField);
setDataURL("ds/test_data/country.data.xml");
setClientOnly(true);
}
}
SmartGWT.zip( 9,880 k)Related examples in the same category