Grid with Custom Grouping Sample (Smart GWT)
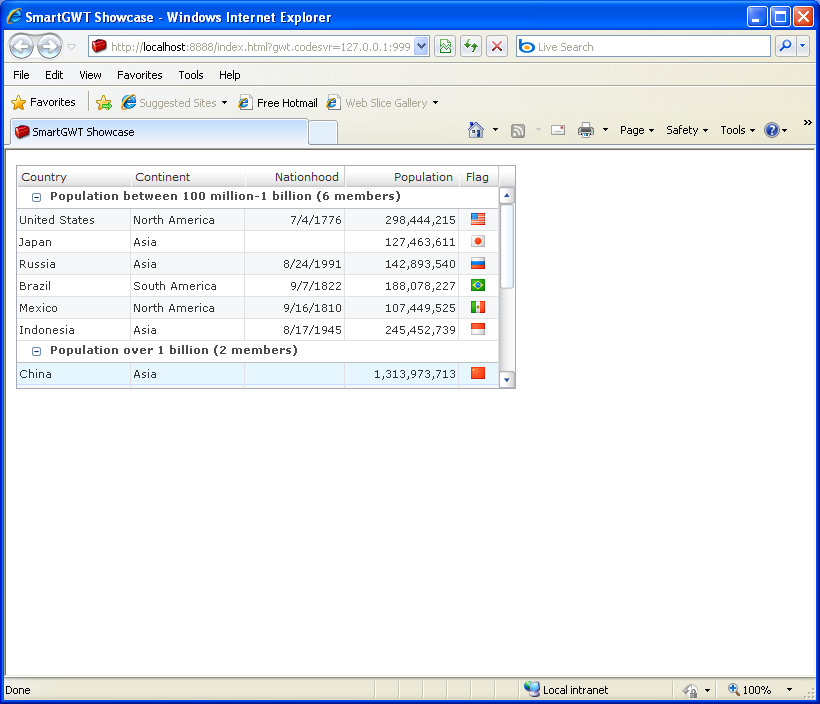
/*
* SmartGWT (GWT for SmartClient)
* Copyright 2008 and beyond, Isomorphic Software, Inc.
*
* SmartGWT is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License version 3
* as published by the Free Software Foundation. SmartGWT is also
* available under typical commercial license terms - see
* http://smartclient.com/license
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*/
package com.smartgwt.sample.showcase.client;
import java.util.Date;
import com.google.gwt.core.client.EntryPoint;
import com.google.gwt.i18n.client.NumberFormat;
import com.google.gwt.user.client.ui.RootPanel;
import com.smartgwt.client.data.DataSource;
import com.smartgwt.client.data.fields.DataSourceBooleanField;
import com.smartgwt.client.data.fields.DataSourceDateField;
import com.smartgwt.client.data.fields.DataSourceFloatField;
import com.smartgwt.client.data.fields.DataSourceIntegerField;
import com.smartgwt.client.data.fields.DataSourceLinkField;
import com.smartgwt.client.data.fields.DataSourceTextField;
import com.smartgwt.client.types.Alignment;
import com.smartgwt.client.types.ListGridFieldType;
import com.smartgwt.client.widgets.Canvas;
import com.smartgwt.client.widgets.grid.CellFormatter;
import com.smartgwt.client.widgets.grid.GroupNode;
import com.smartgwt.client.widgets.grid.GroupTitleRenderer;
import com.smartgwt.client.widgets.grid.GroupValueFunction;
import com.smartgwt.client.widgets.grid.ListGrid;
import com.smartgwt.client.widgets.grid.ListGridField;
import com.smartgwt.client.widgets.grid.ListGridRecord;
public class Showcase implements EntryPoint {
public void onModuleLoad() {
RootPanel.get().add(getViewPanel());
}
public Canvas getViewPanel() {
CountryXmlDS dataSource = CountryXmlDS.getInstance();
final ListGrid countryGrid = new ListGrid();
countryGrid.setCanEdit(true);
countryGrid.setWidth(500);
countryGrid.setHeight(224);
countryGrid.setAlternateRecordStyles(true);
countryGrid.setShowAllRecords(true);
countryGrid.setCellHeight(22);
countryGrid.setDataSource(dataSource);
countryGrid.setGroupStartOpen("all");
countryGrid.setGroupByField("population");
countryGrid.setAutoFetchData(true);
ListGridField nameField = new ListGridField("countryName");
ListGridField continentField = new ListGridField("continent");
ListGridField independenceField = new ListGridField("independence");
independenceField.setGroupValueFunction(new GroupValueFunction() {
public Object getGroupValue(Object value, ListGridRecord record, ListGridField field, String fieldName, ListGrid grid) {
Date independence = (Date) value;
if(independence == null) {
return "Ancient";
} else if (independence.getYear() < 10) { //if year < 1910
return "Pre-Industrial";
} else {
return "Post-Industrial";
}
}
});
final int groupSmall = 1;
final int groupMed = 2;
final int groupLarge = 3;
ListGridField populationField = new ListGridField("population");
populationField.setType(ListGridFieldType.INTEGER);
populationField.setCellFormatter(new CellFormatter() {
public String format(Object value, ListGridRecord record, int rowNum, int colNum) {
if(value == null) return null;
try {
NumberFormat nf = NumberFormat.getFormat("0,000");
return nf.format(((Number) value).longValue());
} catch (Exception e) {
return value.toString();
}
}
});
populationField.setGroupValueFunction(new GroupValueFunction() {
public Object getGroupValue(Object value, ListGridRecord record, ListGridField field, String fieldName, ListGrid grid) {
int population = (Integer) value;
if(population < 100000000) {
return groupSmall;
} else if (population < 1000000000) {
return groupMed;
} else {
return groupLarge;
}
}
});
populationField.setGroupTitleRenderer(new GroupTitleRenderer() {
public String getGroupTitle(Object groupValue, GroupNode groupNode, ListGridField field, String fieldName, ListGrid grid) {
final int groupType = (Integer) groupValue;
String baseTitle ="";
switch (groupType) {
case groupSmall:
baseTitle = "Population less than 100 million";
break;
case groupMed:
baseTitle = "Population between 100 million-1 billion";
break;
case groupLarge:
baseTitle = "Population over 1 billion";
break;
}
baseTitle += " (" + groupNode.getGroupMembers().length + " members)";
return baseTitle;
}
});
ListGridField countryCodeField = new ListGridField("countryCode", "Flag", 40);
countryCodeField.setAlign(Alignment.CENTER);
countryCodeField.setType(ListGridFieldType.IMAGE);
countryCodeField.setImageURLPrefix("flags/16/");
countryCodeField.setImageURLSuffix(".png");
countryCodeField.setCanEdit(false);
countryGrid.setFields(nameField, continentField, independenceField, populationField, countryCodeField);
return countryGrid;
}
}
class CountryXmlDS extends DataSource {
private static CountryXmlDS instance = null;
public static CountryXmlDS getInstance() {
if (instance == null) {
instance = new CountryXmlDS("countryDS");
}
return instance;
}
public CountryXmlDS(String id) {
setID(id);
setRecordXPath("/List/country");
DataSourceIntegerField pkField = new DataSourceIntegerField("pk");
pkField.setHidden(true);
pkField.setPrimaryKey(true);
DataSourceTextField countryCodeField = new DataSourceTextField("countryCode", "Code");
countryCodeField.setRequired(true);
DataSourceTextField countryNameField = new DataSourceTextField("countryName", "Country");
countryNameField.setRequired(true);
DataSourceTextField capitalField = new DataSourceTextField("capital", "Capital");
DataSourceTextField governmentField = new DataSourceTextField("government", "Government", 500);
DataSourceBooleanField memberG8Field = new DataSourceBooleanField("member_g8", "G8");
DataSourceTextField continentField = new DataSourceTextField("continent", "Continent");
continentField.setValueMap("Europe", "Asia", "North America", "Australia/Oceania", "South America", "Africa");
DataSourceDateField independenceField = new DataSourceDateField("independence", "Nationhood");
DataSourceFloatField areaField = new DataSourceFloatField("area", "Area (km&sup2;)");
DataSourceIntegerField populationField = new DataSourceIntegerField("population", "Population");
DataSourceFloatField gdpField = new DataSourceFloatField("gdp", "GDP ($M)");
DataSourceLinkField articleField = new DataSourceLinkField("article", "Info");
setFields(pkField, countryCodeField, countryNameField, capitalField, governmentField,
memberG8Field, continentField, independenceField, areaField, populationField,
gdpField, articleField);
setDataURL("ds/test_data/country.data.xml");
setClientOnly(true);
}
}
SmartGWT.zip( 9,880 k)Related examples in the same category