Print preview (Smart GWT)
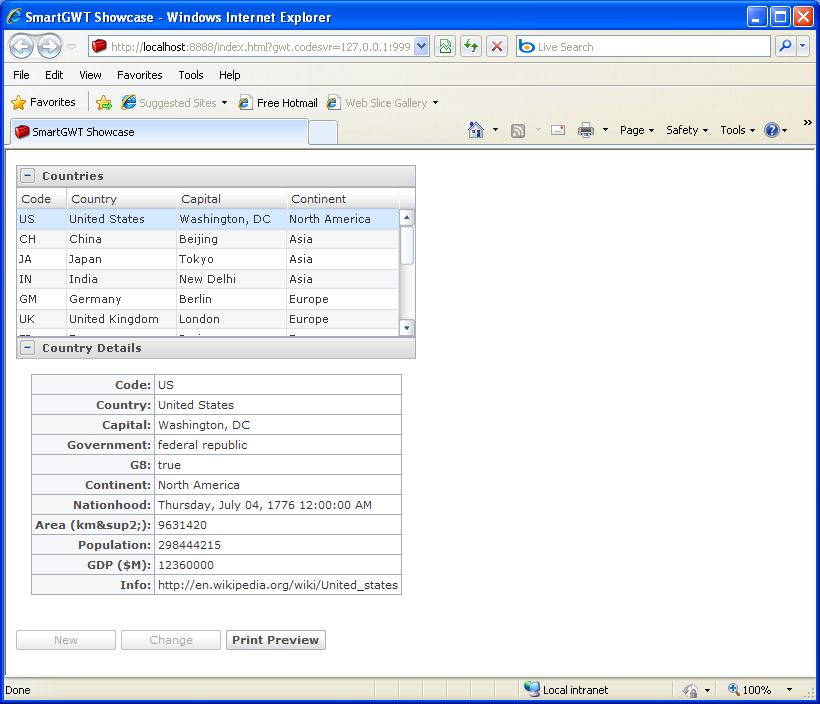
/*
* SmartGWT (GWT for SmartClient)
* Copyright 2008 and beyond, Isomorphic Software, Inc.
*
* SmartGWT is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License version 3
* as published by the Free Software Foundation. SmartGWT is also
* available under typical commercial license terms - see
* http://smartclient.com/license
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*/
package com.smartgwt.sample.showcase.client;
import com.google.gwt.core.client.EntryPoint;
import com.google.gwt.user.client.ui.RootPanel;
import com.smartgwt.client.data.Criteria;
import com.smartgwt.client.data.DSCallback;
import com.smartgwt.client.data.DSRequest;
import com.smartgwt.client.data.DSResponse;
import com.smartgwt.client.data.DataSource;
import com.smartgwt.client.data.Record;
import com.smartgwt.client.data.fields.DataSourceBooleanField;
import com.smartgwt.client.data.fields.DataSourceDateField;
import com.smartgwt.client.data.fields.DataSourceFloatField;
import com.smartgwt.client.data.fields.DataSourceIntegerField;
import com.smartgwt.client.data.fields.DataSourceLinkField;
import com.smartgwt.client.data.fields.DataSourceTextField;
import com.smartgwt.client.types.VisibilityMode;
import com.smartgwt.client.widgets.Canvas;
import com.smartgwt.client.widgets.IButton;
import com.smartgwt.client.widgets.events.ClickEvent;
import com.smartgwt.client.widgets.events.ClickHandler;
import com.smartgwt.client.widgets.grid.ListGrid;
import com.smartgwt.client.widgets.grid.ListGridField;
import com.smartgwt.client.widgets.grid.events.RecordClickEvent;
import com.smartgwt.client.widgets.grid.events.RecordClickHandler;
import com.smartgwt.client.widgets.layout.HLayout;
import com.smartgwt.client.widgets.layout.SectionStack;
import com.smartgwt.client.widgets.layout.SectionStackSection;
import com.smartgwt.client.widgets.layout.VLayout;
import com.smartgwt.client.widgets.viewer.DetailViewer;
public class Showcase implements EntryPoint {
public void onModuleLoad() {
RootPanel.get().add(getViewPanel());
}
public Canvas getViewPanel() {
CountryXmlDS countryDS = CountryXmlDS.getInstance();
SectionStack printStack = new SectionStack();
printStack.setVisibilityMode(VisibilityMode.MULTIPLE);
printStack.setWidth(400);
printStack.setHeight(455);
final DetailViewer printViewer = new DetailViewer();
printViewer.setDataSource(countryDS);
printViewer.setWidth100();
printViewer.setMargin(15);
printViewer.setEmptyMessage("Select a country to view its details");
final ListGrid printGrid = new ListGrid();
printGrid.setHeight(150);
printGrid.setAlternateRecordStyles(true);
printGrid.setDataSource(countryDS);
ListGridField countryCode = new ListGridField("countryCode", "Code", 50);
ListGridField countryName = new ListGridField("countryName", "Country");
ListGridField capital = new ListGridField("capital", "Capital");
ListGridField continent = new ListGridField("continent", "Continent");
printGrid.setFields(countryCode, countryName, capital, continent);
printGrid.addRecordClickHandler(new RecordClickHandler() {
public void onRecordClick(RecordClickEvent event) {
printViewer.setData(new Record[]{event.getRecord()});
}
});
SectionStackSection countriesSection = new SectionStackSection("Countries");
countriesSection.setExpanded(true);
countriesSection.addItem(printGrid);
printStack.addSection(countriesSection);
SectionStackSection detailsSection = new SectionStackSection("Country Details");
detailsSection.setExpanded(true);
detailsSection.addItem(printViewer);
printStack.addSection(detailsSection);
final VLayout printContainer = new VLayout(10);
HLayout printButtonLayout = new HLayout(5);
IButton newButton = new IButton("New");
newButton.setDisabled(true);
IButton changeButton = new IButton("Change");
changeButton.setDisabled(true);
IButton printPreviewButton = new IButton("Print Preview");
printPreviewButton.addClickHandler(new ClickHandler() {
public void onClick(ClickEvent event) {
Canvas.showPrintPreview(printContainer);
}
});
printButtonLayout.addMember(newButton);
printButtonLayout.addMember(changeButton);
printButtonLayout.addMember(printPreviewButton);
printContainer.addMember(printStack);
printContainer.addMember(printButtonLayout);
// The filter is just to limit the number of records in the ListGrid - we don't want to print them all
printGrid.filterData(new Criteria("CountryName", "land"), new DSCallback() {
public void execute(DSResponse response, Object rawData, DSRequest request) {
printGrid.selectRecord(0);
printViewer.setData(new Record[]{printGrid.getSelectedRecord()});
}
});
return printContainer;
}
}
class CountryXmlDS extends DataSource {
private static CountryXmlDS instance = null;
public static CountryXmlDS getInstance() {
if (instance == null) {
instance = new CountryXmlDS("countryDS");
}
return instance;
}
public CountryXmlDS(String id) {
setID(id);
setRecordXPath("/List/country");
DataSourceIntegerField pkField = new DataSourceIntegerField("pk");
pkField.setHidden(true);
pkField.setPrimaryKey(true);
DataSourceTextField countryCodeField = new DataSourceTextField("countryCode", "Code");
countryCodeField.setRequired(true);
DataSourceTextField countryNameField = new DataSourceTextField("countryName", "Country");
countryNameField.setRequired(true);
DataSourceTextField capitalField = new DataSourceTextField("capital", "Capital");
DataSourceTextField governmentField = new DataSourceTextField("government", "Government", 500);
DataSourceBooleanField memberG8Field = new DataSourceBooleanField("member_g8", "G8");
DataSourceTextField continentField = new DataSourceTextField("continent", "Continent");
continentField.setValueMap("Europe", "Asia", "North America", "Australia/Oceania",
"South America", "Africa");
DataSourceDateField independenceField = new DataSourceDateField("independence", "Nationhood");
DataSourceFloatField areaField = new DataSourceFloatField("area", "Area (km²)");
DataSourceIntegerField populationField = new DataSourceIntegerField("population", "Population");
DataSourceFloatField gdpField = new DataSourceFloatField("gdp", "GDP ($M)");
DataSourceLinkField articleField = new DataSourceLinkField("article", "Info");
setFields(pkField, countryCodeField, countryNameField, capitalField, governmentField,
memberG8Field, continentField, independenceField, areaField, populationField, gdpField,
articleField);
setDataURL("ds/test_data/country.data.xml");
setClientOnly(true);
}
}
SmartGWT.zip( 9,880 k)Related examples in the same category