BreakIterator for difference locales
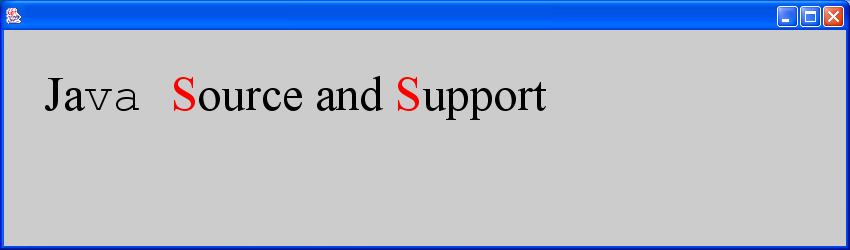
import java.awt.Component;
import java.awt.Dimension;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.text.BreakIterator;
import java.util.Locale;
import java.util.Vector;
import javax.swing.ButtonGroup;
import javax.swing.DefaultListCellRenderer;
import javax.swing.JButton;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JList;
import javax.swing.JPanel;
import javax.swing.JRadioButton;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
public class IteratorTest extends JPanel {
protected JComboBox localeButton = new JComboBox(Locale.getAvailableLocales());
protected JTextArea textArea = new JTextArea(5, 20);
protected JRadioButton charButton = new JRadioButton("Character", true);
protected JRadioButton wordButton = new JRadioButton("Word");
protected JRadioButton lineButton = new JRadioButton("Line");
protected JRadioButton sentButton = new JRadioButton("Sentence");
protected JButton refreshButton = new JButton("Refresh");
protected JList itemList = new JList();
public static void main(String[] args) {
JFrame f = new JFrame();
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setContentPane(new IteratorTest());
f.pack();
f.setVisible(true);
}
public IteratorTest() {
localeButton.setRenderer(new LocaleListCellRenderer());
localeButton.setSelectedItem(Locale.getDefault());
add(localeButton);
textArea.setLineWrap(true);
textArea.setWrapStyleWord(true);
add(new JScrollPane(textArea));
add(getTypePanel());
add(getCountPanel());
add(new JScrollPane(itemList));
refreshDisplay();
}
protected JPanel getTypePanel() {
JPanel panel = new JPanel();
panel.add(charButton);
panel.add(wordButton);
panel.add(lineButton);
panel.add(sentButton);
ButtonGroup group = new ButtonGroup();
group.add(charButton);
group.add(wordButton);
group.add(lineButton);
group.add(sentButton);
return panel;
}
protected JPanel getCountPanel() {
JPanel panel = new JPanel();
JLabel label = new JLabel("Count:", JLabel.RIGHT);
panel.add(label);
Dimension size = panel.getPreferredSize();
size.width = Math.min(size.width, 100);
refreshButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent event) {
refreshDisplay();
}
});
panel.add(refreshButton);
return panel;
}
protected void refreshDisplay() {
int startIndex, nextIndex;
Vector items = new Vector();
String msgText = textArea.getText();
Locale locale = (Locale) (localeButton.getSelectedItem());
BreakIterator iterator = null;
if (charButton.isSelected()) {
iterator = BreakIterator.getCharacterInstance(locale);
} else if (wordButton.isSelected()) {
iterator = BreakIterator.getWordInstance(locale);
} else if (lineButton.isSelected()) {
iterator = BreakIterator.getLineInstance(locale);
} else if (sentButton.isSelected()) {
iterator = BreakIterator.getSentenceInstance(locale);
}
iterator.setText(msgText);
startIndex = iterator.first();
nextIndex = iterator.next();
while (nextIndex != BreakIterator.DONE) {
items.addElement(msgText.substring(startIndex, nextIndex));
startIndex = nextIndex;
nextIndex = iterator.next();
}
itemList.setListData(items);
}
}
class LocaleListCellRenderer extends DefaultListCellRenderer {
public Component getListCellRendererComponent(JList list, Object value, int index,
boolean isSelected, boolean hasFocus) {
Locale locale = (Locale) (value);
return super.getListCellRendererComponent(list, locale.getDisplayName(), index, isSelected,
hasFocus);
}
}
Related examples in the same category