Displaying Formatted Numbers for Alternate Locales
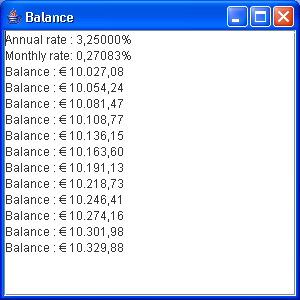
import java.math.BigDecimal;
import java.text.DecimalFormat;
import java.text.NumberFormat;
import java.util.Locale;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
public class BigDecFormat {
public static void main(String args[]) {
StringBuffer buffer = new StringBuffer();
Locale italian = new Locale("it", "IT", "EURO");
Locale.setDefault(italian);
BigDecimal rate = new BigDecimal(".03250000");
BigDecimal months = new BigDecimal("12");
BigDecimal monthlyRate = rate
.divide(months, BigDecimal.ROUND_HALF_DOWN);
NumberFormat pf = new DecimalFormat("#,##0.00000%");
buffer.append("Annual rate : " + pf.format(rate.doubleValue()) + "\n");
buffer.append("Monthly rate: " + pf.format(monthlyRate.doubleValue())
+ "\n");
BigDecimal balance = new BigDecimal("10000.0000");
NumberFormat nf = NumberFormat.getCurrencyInstance();
for (int i = 0; i < 12; i++) {
BigDecimal interest = balance.multiply(monthlyRate);
balance = balance.add(interest);
buffer.append("Balance : " + nf.format(balance.doubleValue())
+ "\n");
}
JFrame frame = new JFrame("Balance");
JTextArea text = new JTextArea(buffer.toString());
JScrollPane pane = new JScrollPane(text);
frame.getContentPane().add(pane);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300, 300);
frame.show();
}
}
Related examples in the same category