StringItem Alternative
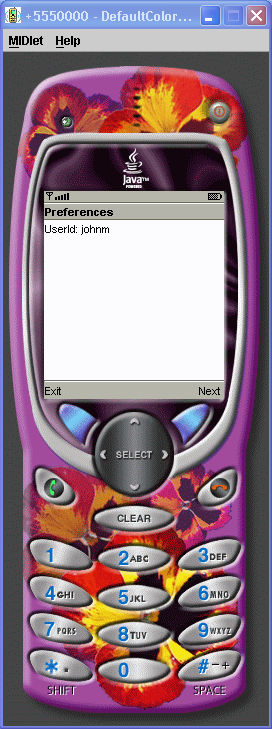
/*--------------------------------------------------
* StringItemAlternative.java
*
* Example from the book: Core J2ME Technology
* Copyright John W. Muchow http://www.CoreJ2ME.com
* You may use/modify for any non-commercial purpose
*-------------------------------------------------*/
import javax.microedition.midlet.*;
import javax.microedition.lcdui.*;
public class StringItemAlternative extends MIDlet implements CommandListener
{
private Display display; // Reference to Display object for this MIDlet
private Form fmMain; // The main form
private Command cmNext; // Next label and message
private Command cmExit; // Command to exit the MIDlet
private int msgIndex; // Index of our message text on form
private static int count = 0; // How many times through our loop
public StringItemAlternative()
{
display = Display.getDisplay(this);
// Create commands
cmNext = new Command("Next", Command.SCREEN, 1);
cmExit = new Command("Exit", Command.EXIT, 1);
// Create Form, add Command & message, listen for events
fmMain = new Form("Preferences");
fmMain.addCommand(cmExit);
fmMain.addCommand(cmNext);
// Save the index location of this item
msgIndex = fmMain.append("UserId: johnm");
fmMain.setCommandListener(this);
}
// Called by application manager to start the MIDlet.
public void startApp()
{
display.setCurrent(fmMain);
}
public void pauseApp()
{ }
public void destroyApp(boolean unconditional)
{ }
public void commandAction(Command c, Displayable s)
{
if (c == cmNext)
{
if (count++ == 0)
{
//--------------------------------
// Option # 1
// First time through this method
//--------------------------------
StringItem tmpItem = (StringItem) fmMain.get(msgIndex);
System.out.println("tmpItem.getLabel(): " + tmpItem.getLabel());
System.out.println("tmpItem.getText(): " + tmpItem.getText());
tmpItem.setLabel("Account #: "); //inherited from Item class
tmpItem.setText("731");
}
else
{
//--------------------------------
// Option # 2
// Second time through this method
//--------------------------------
fmMain.set(msgIndex, new StringItem("Password: ", "superPants"));
// Remove the Update command
fmMain.removeCommand(cmNext);
}
}
else if (c == cmExit)
{
destroyApp(false);
notifyDestroyed();
}
}
}
Related examples in the same category