Creating Chapter Section in PDF document
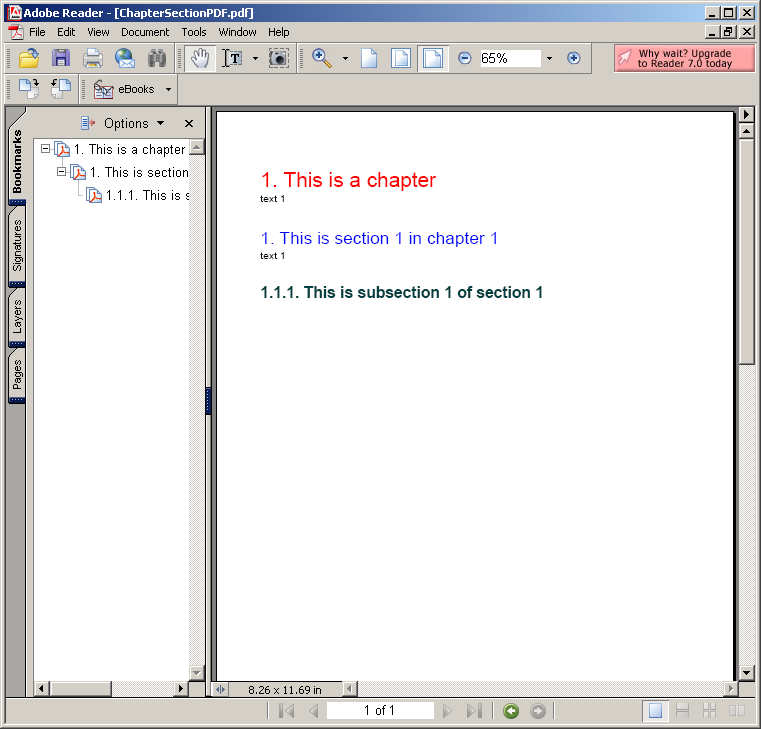
import java.awt.Color;
import java.io.FileOutputStream;
import com.lowagie.text.Chapter;
import com.lowagie.text.Document;
import com.lowagie.text.Element;
import com.lowagie.text.Font;
import com.lowagie.text.FontFactory;
import com.lowagie.text.PageSize;
import com.lowagie.text.Paragraph;
import com.lowagie.text.Section;
import com.lowagie.text.pdf.PdfWriter;
public class ChapterSectionPDF {
public static void main(String[] args) {
Document document = new Document(PageSize.A4, 50, 50, 50, 50);
try {
PdfWriter writer = PdfWriter.getInstance(document, new FileOutputStream("ChapterSectionPDF.pdf"));
writer.setViewerPreferences(PdfWriter.PageModeUseOutlines);
document.open();
Font chapterFont = FontFactory.getFont(FontFactory.HELVETICA, 24, Font.NORMAL, new Color(255, 0, 0));
Font sectionFont = FontFactory.getFont(FontFactory.HELVETICA, 20, Font.NORMAL, new Color(0, 0, 255));
Font subsectionFont = FontFactory.getFont(FontFactory.HELVETICA, 18, Font.BOLD, new Color(0, 64, 64));
Paragraph paragraph1 = new Paragraph("text 1");
Paragraph paragraph2 = new Paragraph("text 2");
Paragraph cTitle = new Paragraph("This is a chapter ", chapterFont);
Chapter chapter = new Chapter(cTitle, 1);
paragraph2.setAlignment(Element.ALIGN_JUSTIFIED);
paragraph1.setAlignment(Element.ALIGN_JUSTIFIED);
chapter.add(paragraph1);
Paragraph sTitle = new Paragraph("This is section 1 in chapter 1" , sectionFont);
Section section = chapter.addSection(sTitle, 1);
section.add(paragraph1);
Paragraph subTitle = new Paragraph("This is subsection 1 of section 1", subsectionFont);
Section subsection = section.addSection(subTitle, 3);
document.add(chapter);
} catch (Exception de) {
de.printStackTrace();
}
document.close();
}
}
itext.zip( 1,748 k)Related examples in the same category