Prevent Tab from traversing out of a control
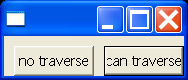
/*
* Control example snippet: prevent Tab from traversing out of a control
*
* For a list of all SWT example snippets see
* http://dev.eclipse.org/viewcvs/index.cgi/%7Echeckout%7E/platform-swt-home/dev.html#snippets
*/
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.TraverseEvent;
import org.eclipse.swt.events.TraverseListener;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
public class Snippet127 {
public static void main (String [] args) {
Display display = new Display ();
Shell shell = new Shell (display);
Button button1 = new Button(shell, SWT.PUSH);
button1.setBounds(10,10,80,30);
button1.setText("no traverse");
button1.addTraverseListener(new TraverseListener () {
public void keyTraversed(TraverseEvent e) {
switch (e.detail) {
case SWT.TRAVERSE_TAB_NEXT:
case SWT.TRAVERSE_TAB_PREVIOUS: {
e.doit = false;
}
}
}
});
Button button2 = new Button (shell, SWT.PUSH);
button2.setBounds(100,10,80,30);
button2.setText("can traverse");
shell.pack ();
shell.open();
while (!shell.isDisposed ()) {
if (!display.readAndDispatch ()) display.sleep ();
}
display.dispose ();
}
}
Related examples in the same category