Encrypting and Decrypting with the JCE
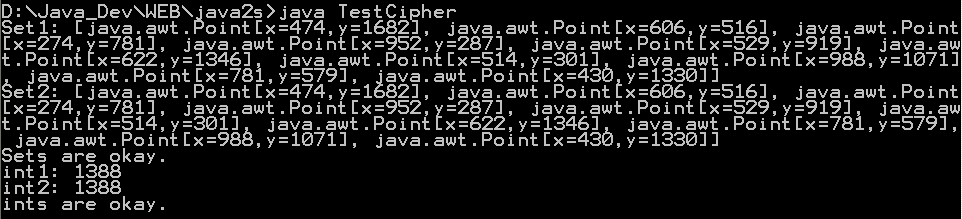
import java.awt.Point;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.util.HashSet;
import java.util.Random;
import java.util.Set;
import javax.crypto.Cipher;
import javax.crypto.CipherInputStream;
import javax.crypto.CipherOutputStream;
import javax.crypto.SecretKey;
import javax.crypto.SecretKeyFactory;
import javax.crypto.spec.DESKeySpec;
public class TestCipher {
// Password must be at least 8 characters
private static final String password = "zukowski";
public static void main(String args[]) throws Exception {
Set set = new HashSet();
Random random = new Random();
for (int i = 0; i < 10; i++) {
Point point = new Point(random.nextInt(1000), random.nextInt(2000));
set.add(point);
}
int last = random.nextInt(5000);
// Create Key
byte key[] = password.getBytes();
DESKeySpec desKeySpec = new DESKeySpec(key);
SecretKeyFactory keyFactory = SecretKeyFactory.getInstance("DES");
SecretKey secretKey = keyFactory.generateSecret(desKeySpec);
// Create Cipher
Cipher desCipher = Cipher.getInstance("DES/ECB/PKCS5Padding");
desCipher.init(Cipher.ENCRYPT_MODE, secretKey);
// Create stream
FileOutputStream fos = new FileOutputStream("out.des");
BufferedOutputStream bos = new BufferedOutputStream(fos);
CipherOutputStream cos = new CipherOutputStream(bos, desCipher);
ObjectOutputStream oos = new ObjectOutputStream(cos);
// Write objects
oos.writeObject(set);
oos.writeInt(last);
oos.flush();
oos.close();
// Change cipher mode
desCipher.init(Cipher.DECRYPT_MODE, secretKey);
// Create stream
FileInputStream fis = new FileInputStream("out.des");
BufferedInputStream bis = new BufferedInputStream(fis);
CipherInputStream cis = new CipherInputStream(bis, desCipher);
ObjectInputStream ois = new ObjectInputStream(cis);
// Read objects
Set set2 = (Set) ois.readObject();
int last2 = ois.readInt();
ois.close();
// Compare original with what was read back
int count = 0;
if (set.equals(set2)) {
System.out.println("Set1: " + set);
System.out.println("Set2: " + set2);
System.out.println("Sets are okay.");
count++;
}
if (last == last2) {
System.out.println("int1: " + last);
System.out.println("int2: " + last2);
System.out.println("ints are okay.");
count++;
}
if (count != 2) {
System.out.println("Problem during encryption/decryption");
}
}
}
Related examples in the same category