A frame with a sample text label and radio buttons for selecting font sizes
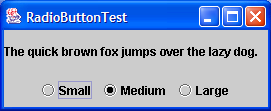
/*
This program is a part of the companion code for Core Java 8th ed.
(http://horstmann.com/corejava)
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not, see <http://www.gnu.org/licenses/>.
*/
import java.awt.BorderLayout;
import java.awt.EventQueue;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.ButtonGroup;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JRadioButton;
/**
* @version 1.33 2007-06-12
* @author Cay Horstmann
*/
public class RadioButtonTest
{
public static void main(String[] args)
{
EventQueue.invokeLater(new Runnable()
{
public void run()
{
RadioButtonFrame frame = new RadioButtonFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
});
}
}
/**
* A frame with a sample text label and radio buttons for selecting font sizes.
*/
class RadioButtonFrame extends JFrame
{
public RadioButtonFrame()
{
setTitle("RadioButtonTest");
setSize(DEFAULT_WIDTH, DEFAULT_HEIGHT);
// add the sample text label
label = new JLabel("The quick brown fox jumps over the lazy dog.");
label.setFont(new Font("Serif", Font.PLAIN, DEFAULT_SIZE));
add(label, BorderLayout.CENTER);
// add the radio buttons
buttonPanel = new JPanel();
group = new ButtonGroup();
addRadioButton("Small", 8);
addRadioButton("Medium", 12);
addRadioButton("Large", 18);
addRadioButton("Extra large", 36);
add(buttonPanel, BorderLayout.SOUTH);
}
/**
* Adds a radio button that sets the font size of the sample text.
* @param name the string to appear on the button
* @param size the font size that this button sets
*/
public void addRadioButton(String name, final int size)
{
boolean selected = size == DEFAULT_SIZE;
JRadioButton button = new JRadioButton(name, selected);
group.add(button);
buttonPanel.add(button);
// this listener sets the label font size
ActionListener listener = new ActionListener()
{
public void actionPerformed(ActionEvent event)
{
// size refers to the final parameter of the addRadioButton
// method
label.setFont(new Font("Serif", Font.PLAIN, size));
}
};
button.addActionListener(listener);
}
public static final int DEFAULT_WIDTH = 400;
public static final int DEFAULT_HEIGHT = 200;
private JPanel buttonPanel;
private ButtonGroup group;
private JLabel label;
private static final int DEFAULT_SIZE = 12;
}
Related examples in the same category