An implementation of JSpinner with customized content--icons
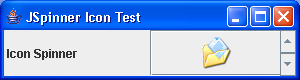
/*
Java Swing, 2nd Edition
By Marc Loy, Robert Eckstein, Dave Wood, James Elliott, Brian Cole
ISBN: 0-596-00408-7
Publisher: O'Reilly
*/
// IconSpinner.java
//An implementation of JSpinner with customized content--icons in this case.
//A standard spinner model is used with a custom editor (IconEditor.java).
//
import java.awt.Container;
import java.awt.GridLayout;
import javax.swing.Icon;
import javax.swing.ImageIcon;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JSpinner;
import javax.swing.SpinnerListModel;
import javax.swing.event.ChangeEvent;
import javax.swing.event.ChangeListener;
public class IconSpinner extends JFrame {
public IconSpinner() {
super("JSpinner Icon Test");
setSize(300, 80);
setDefaultCloseOperation(EXIT_ON_CLOSE);
Container c = getContentPane();
c.setLayout(new GridLayout(0, 2));
Icon nums[] = new Icon[] { new ImageIcon("1.gif"),
new ImageIcon("2.gif"), new ImageIcon("3.gif"),
new ImageIcon("4.gif"), new ImageIcon("5.gif"),
new ImageIcon("6.gif") };
JSpinner s1 = new JSpinner(new SpinnerListModel(nums));
s1.setEditor(new IconEditor(s1));
c.add(new JLabel(" Icon Spinner"));
c.add(s1);
setVisible(true);
}
public static void main(String args[]) {
new IconSpinner();
}
}
class IconEditor extends JLabel implements ChangeListener {
JSpinner spinner;
Icon icon;
public IconEditor(JSpinner s) {
super((Icon) s.getValue(), CENTER);
icon = (Icon) s.getValue();
spinner = s;
spinner.addChangeListener(this);
}
public void stateChanged(ChangeEvent ce) {
icon = (Icon) spinner.getValue();
setIcon(icon);
}
public JSpinner getSpinner() {
return spinner;
}
public Icon getIcon() {
return icon;
}
}
Related examples in the same category