Demonstrates JoptionPane
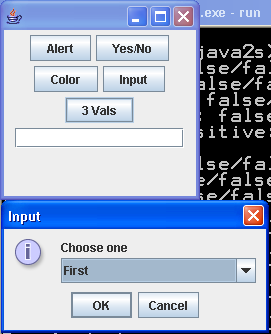
// : c14:MessageBoxes.java
// Demonstrates JoptionPane.
// <applet code=MessageBoxes width=200 height=150></applet>
// From 'Thinking in Java, 3rd ed.' (c) Bruce Eckel 2002
// www.BruceEckel.com. See copyright notice in CopyRight.txt.
import java.awt.Container;
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JApplet;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JTextField;
public class MessageBoxes extends JApplet {
private JButton[] b = { new JButton("Alert"), new JButton("Yes/No"),
new JButton("Color"), new JButton("Input"), new JButton("3 Vals") };
private JTextField txt = new JTextField(15);
private ActionListener al = new ActionListener() {
public void actionPerformed(ActionEvent e) {
String id = ((JButton) e.getSource()).getText();
if (id.equals("Alert"))
JOptionPane.showMessageDialog(null, "There's a bug on you!",
"Hey!", JOptionPane.ERROR_MESSAGE);
else if (id.equals("Yes/No"))
JOptionPane.showConfirmDialog(null, "or no", "choose yes",
JOptionPane.YES_NO_OPTION);
else if (id.equals("Color")) {
Object[] options = { "Red", "Green" };
int sel = JOptionPane.showOptionDialog(null, "Choose a Color!",
"Warning", JOptionPane.DEFAULT_OPTION,
JOptionPane.WARNING_MESSAGE, null, options, options[0]);
if (sel != JOptionPane.CLOSED_OPTION)
txt.setText("Color Selected: " + options[sel]);
} else if (id.equals("Input")) {
String val = JOptionPane
.showInputDialog("How many fingers do you see?");
txt.setText(val);
} else if (id.equals("3 Vals")) {
Object[] selections = { "First", "Second", "Third" };
Object val = JOptionPane.showInputDialog(null, "Choose one",
"Input", JOptionPane.INFORMATION_MESSAGE, null,
selections, selections[0]);
if (val != null)
txt.setText(val.toString());
}
}
};
public void init() {
Container cp = getContentPane();
cp.setLayout(new FlowLayout());
for (int i = 0; i < b.length; i++) {
b[i].addActionListener(al);
cp.add(b[i]);
}
cp.add(txt);
}
public static void main(String[] args) {
run(new MessageBoxes(), 200, 200);
}
public static void run(JApplet applet, int width, int height) {
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().add(applet);
frame.setSize(width, height);
applet.init();
applet.start();
frame.setVisible(true);
}
} ///:~
Related examples in the same category