Property Split
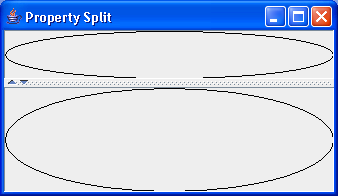
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Container;
import java.awt.Graphics;
import java.awt.GridLayout;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import javax.swing.JComponent;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JSplitPane;
public class PropertySplit {
public static void main(String args[]) {
JFrame frame = new JFrame("Property Split");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JSplitPane splitPane = new JSplitPane(JSplitPane.VERTICAL_SPLIT);
splitPane.setContinuousLayout(true);
splitPane.setOneTouchExpandable(true);
JComponent topComponent = new OvalPanel();
splitPane.setTopComponent(topComponent);
JComponent bottomComponent = new OvalPanel();
splitPane.setBottomComponent(bottomComponent);
PropertyChangeListener propertyChangeListener = new PropertyChangeListener() {
public void propertyChange(PropertyChangeEvent changeEvent) {
JSplitPane sourceSplitPane = (JSplitPane) changeEvent
.getSource();
String propertyName = changeEvent.getPropertyName();
if (propertyName
.equals(JSplitPane.LAST_DIVIDER_LOCATION_PROPERTY)) {
int current = sourceSplitPane.getDividerLocation();
System.out.println("Current: " + current);
Integer last = (Integer) changeEvent.getNewValue();
System.out.println("Last: " + last);
Integer priorLast = (Integer) changeEvent.getOldValue();
System.out.println("Prior last: " + priorLast);
}
}
};
splitPane.addPropertyChangeListener(propertyChangeListener);
Container contentPane = frame.getContentPane();
contentPane.add(splitPane, BorderLayout.CENTER);
frame.setSize(300, 150);
frame.setVisible(true);
}
}
class OvalPanel extends JPanel {
Color color;
public OvalPanel() {
this(Color.black);
}
public OvalPanel(Color color) {
this.color = color;
}
public void paintComponent(Graphics g) {
int width = getWidth();
int height = getHeight();
g.setColor(color);
g.drawOval(0, 0, width, height);
}
}
Related examples in the same category