Working with Toggle Buttons
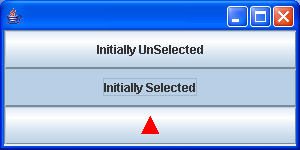
import java.awt.Color;
import java.awt.Component;
import java.awt.Container;
import java.awt.Graphics;
import java.awt.GridLayout;
import java.awt.Polygon;
import javax.swing.Icon;
import javax.swing.JFrame;
import javax.swing.JToggleButton;
public class ToggleTest {
static class TriangleIcon implements Icon {
String name;
static class State {
public static final int NORMAL = 0;
public static final int PRESSED = 1;
public static final int ROLLOVER = 2;
public static final int SELECTED = 3;
private State() {
}
}
int state;
Color color;
public TriangleIcon(Color c, int state) {
color = c;
this.state = state;
}
public int getIconWidth() {
return 20;
}
public int getIconHeight() {
return 20;
}
public void paintIcon(Component c, Graphics g, int x, int y) {
g.setColor(color);
Polygon p = new Polygon();
if (state == State.NORMAL) {
p.addPoint(x + (getIconWidth() / 2), y);
p.addPoint(x, y + getIconHeight() - 1);
p.addPoint(x + getIconWidth() - 1, y + getIconHeight() - 1);
} else if (state == State.PRESSED) {
p.addPoint(x, y);
p.addPoint(x + getIconWidth() - 1, y);
p.addPoint(x + (getIconWidth() / 2), y + getIconHeight() - 1);
} else if (state == State.SELECTED) {
p.addPoint(x + getIconWidth() - 1, y);
p.addPoint(x + getIconWidth() - 1, y + getIconHeight() - 1);
p.addPoint(x, y + (getIconHeight() / 2));
} else {
p.addPoint(x, y);
p.addPoint(x, y + getIconHeight() - 1);
p.addPoint(x + getIconWidth() - 1, y + (getIconHeight() / 2));
}
g.fillPolygon(p);
}
}
public static void main(String args[]) {
JFrame frame = new JFrame();
Container contentPane = frame.getContentPane();
contentPane.setLayout(new GridLayout(0, 1));
Icon normalIcon = new TriangleIcon(Color.red, TriangleIcon.State.NORMAL);
Icon pressedIcon = new TriangleIcon(Color.red,
TriangleIcon.State.PRESSED);
Icon selectedIcon = new TriangleIcon(Color.red,
TriangleIcon.State.SELECTED);
Icon rolloverIcon = new TriangleIcon(Color.red,
TriangleIcon.State.ROLLOVER);
JToggleButton b = new JToggleButton("Initially UnSelected");
contentPane.add(b);
b = new JToggleButton("Initially Selected", true);
contentPane.add(b);
b = new JToggleButton(normalIcon);
b.setPressedIcon(pressedIcon);
b.setSelectedIcon(selectedIcon);
b.setRolloverIcon(rolloverIcon);
b.setRolloverEnabled(true);
contentPane.add(b);
frame.setSize(300, 150);
frame.show();
}
}
Related examples in the same category