Demo Tree Walker
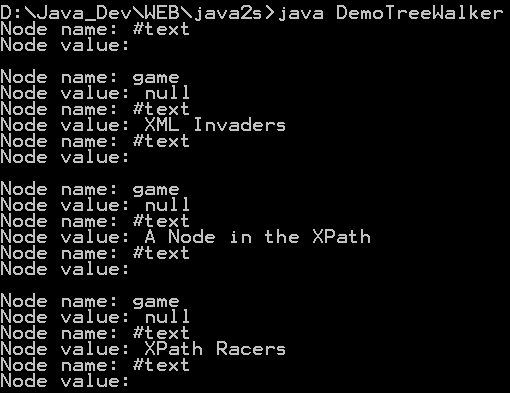
/*
Java, XML, and Web Services Bible
Mike Jasnowski
ISBN: 0-7645-4847-6
*/
import org.apache.xerces.parsers.DOMParser;
import org.xml.sax.SAXException;
import org.w3c.dom.traversal.DocumentTraversal;
import org.w3c.dom.Document;
import org.w3c.dom.Node;
import java.io.IOException;
import org.w3c.dom.traversal.NodeFilter;
import org.w3c.dom.traversal.TreeWalker;
public class DemoTreeWalker {
public static void main(String args[]) throws SAXException,IOException{
DOMParser parser = new DOMParser();
parser.parse("games.xml");
Document doc = parser.getDocument();
DocumentTraversal docTraversal = (DocumentTraversal)doc;
TreeWalker iter =
docTraversal.createTreeWalker(doc.getDocumentElement(),
NodeFilter.SHOW_ALL,
null,
false);
Node n = null;
while ((n=iter.nextNode())!=null){
System.out.println("Node name: " +
n.getNodeName());
System.out.println("Node value: " +
n.getNodeValue());
}
}
}
//game.xml
/*
<?xml version="1.0"?>
<games>
<game genre="shooter">XML Invaders</game>
<game genre="rpg">A Node in the XPath</game>
<game genre="action">XPath Racers</game>
</games>
*/
Related examples in the same category