Parse Properties Files
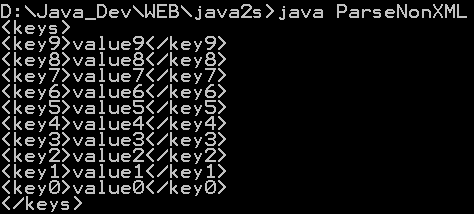
import org.xml.sax.*;
import org.xml.sax.helpers.*;
import java.util.Properties;
import org.xml.sax.*;
import org.xml.sax.helpers.*;
import java.util.Properties;
import java.util.Enumeration;
import org.apache.xerces.parsers.*;
public class ParseNonXML extends DefaultHandler {
public static void main(String args[]) throws SAXException {
PropertyFileParser pfp = new PropertyFileParser();
pfp.setContentHandler(new ParseNonXML());
pfp.parse(buildProperties());
}
public static Properties buildProperties() {
Properties props = new Properties();
for (int i = 0; i < 10; i++)
props.setProperty("key" + i, "value" + i);
return props;
}
public void startDocument() {
System.out.println("<keys>");
}
public void endDocument() {
System.out.println("</keys>");
}
public void characters(char[] data, int start, int end) {
String str = new String(data, start, end);
System.out.print(str);
}
public void startElement(String uri, String qName, String lName, Attributes atts) {
System.out.print("<" + lName + ">");
}
public void endElement(String uri, String qName, String lName) {
System.out.println("</" + lName + ">");
}
}
class PropertyFileParser extends SAXParser {
private Properties props = null;
private ContentHandler handler = null;
public void parse(Properties props) throws SAXException {
handler = getContentHandler();
handler.startDocument();
Enumeration e = props.propertyNames();
while (e.hasMoreElements()) {
String key = (String) e.nextElement();
String val = (String) props.getProperty(key);
handler.startElement("", key, key, new AttributesImpl());
char[] chars = getChars(val);
handler.characters(chars, 0, chars.length);
handler.endElement("", key, key);
}
handler.endDocument();
}
private char[] getChars(String value) {
char[] chars = new char[value.length()];
value.getChars(0, value.length(), chars, 0);
return chars;
}
}
Related examples in the same category