XSL transformations: It applies a transformation to a set of employee records
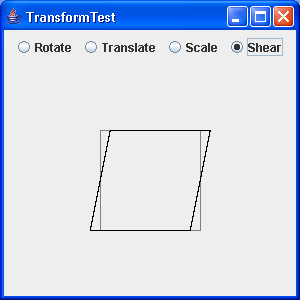
/*
This program is a part of the companion code for Core Java 8th ed.
(http://horstmann.com/corejava)
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not, see <http://www.gnu.org/licenses/>.
*/
import java.io.*;
import java.util.*;
import javax.xml.transform.*;
import javax.xml.transform.sax.*;
import javax.xml.transform.stream.*;
import org.xml.sax.*;
import org.xml.sax.helpers.*;
/**
* This program demonstrates XSL transformations. It applies a transformation to a set of employee
* records. The records are stored in the file employee.dat and turned into XML format. Specify the
* stylesheet on the command line, e.g. java TransformTest makeprop.xsl
* @version 1.01 2007-06-25
* @author Cay Horstmann
*/
public class TransformTest
{
public static void main(String[] args) throws Exception
{
String filename;
if (args.length > 0) filename = args[0];
else filename = "makehtml.xsl";
File styleSheet = new File(filename);
StreamSource styleSource = new StreamSource(styleSheet);
Transformer t = TransformerFactory.newInstance().newTransformer(styleSource);
t.setOutputProperty(OutputKeys.INDENT, "yes");
t.setOutputProperty(OutputKeys.METHOD, "xml");
t.setOutputProperty("{http://xml.apache.org/xslt}indent-amount", "2");
t.transform(new SAXSource(new EmployeeReader(), new InputSource(new FileInputStream(
"employee.dat"))), new StreamResult(System.out));
}
}
/**
* This class reads the flat file employee.dat and reports SAX parser events to act as if it was
* parsing an XML file.
*/
class EmployeeReader implements XMLReader
{
public void parse(InputSource source) throws IOException, SAXException
{
InputStream stream = source.getByteStream();
BufferedReader in = new BufferedReader(new InputStreamReader(stream));
String rootElement = "staff";
AttributesImpl atts = new AttributesImpl();
if (handler == null) throw new SAXException("No content handler");
handler.startDocument();
handler.startElement("", rootElement, rootElement, atts);
String line;
while ((line = in.readLine()) != null)
{
handler.startElement("", "employee", "employee", atts);
StringTokenizer t = new StringTokenizer(line, "|");
handler.startElement("", "name", "name", atts);
String s = t.nextToken();
handler.characters(s.toCharArray(), 0, s.length());
handler.endElement("", "name", "name");
handler.startElement("", "salary", "salary", atts);
s = t.nextToken();
handler.characters(s.toCharArray(), 0, s.length());
handler.endElement("", "salary", "salary");
atts.addAttribute("", "year", "year", "CDATA", t.nextToken());
atts.addAttribute("", "month", "month", "CDATA", t.nextToken());
atts.addAttribute("", "day", "day", "CDATA", t.nextToken());
handler.startElement("", "hiredate", "hiredate", atts);
handler.endElement("", "hiredate", "hiredate");
atts.clear();
handler.endElement("", "employee", "employee");
}
handler.endElement("", rootElement, rootElement);
handler.endDocument();
}
public void setContentHandler(ContentHandler newValue)
{
handler = newValue;
}
public ContentHandler getContentHandler()
{
return handler;
}
// the following methods are just do-nothing implementations
public void parse(String systemId) throws IOException, SAXException
{
}
public void setErrorHandler(ErrorHandler handler)
{
}
public ErrorHandler getErrorHandler()
{
return null;
}
public void setDTDHandler(DTDHandler handler)
{
}
public DTDHandler getDTDHandler()
{
return null;
}
public void setEntityResolver(EntityResolver resolver)
{
}
public EntityResolver getEntityResolver()
{
return null;
}
public void setProperty(String name, Object value)
{
}
public Object getProperty(String name)
{
return null;
}
public void setFeature(String name, boolean value)
{
}
public boolean getFeature(String name)
{
return false;
}
private ContentHandler handler;
}
//File: makehtml.xsl
<?xml version="1.0" encoding="ISO-8859-1"?>
<xsl:stylesheet
xmlns:xsl="http://www.w3.org/1999/XSL/Transform"
version="1.0">
<xsl:output method="html"/>
<xsl:template match="/staff">
<table border="1"><xsl:apply-templates/></table>
</xsl:template>
<xsl:template match="/staff/employee">
<tr><xsl:apply-templates/></tr>
</xsl:template>
<xsl:template match="/staff/employee/name">
<td><xsl:apply-templates/></td>
</xsl:template>
<xsl:template match="/staff/employee/salary">
<td>$<xsl:apply-templates/></td>
</xsl:template>
<xsl:template match="/staff/employee/hiredate">
<td><xsl:value-of select="@year"/>-<xsl:value-of
select="@month"/>-<xsl:value-of select="@day"/></td>
</xsl:template>
</xsl:stylesheet>
//File: makeprop.xsl
<?xml version="1.0"?>
<xsl:stylesheet
xmlns:xsl="http://www.w3.org/1999/XSL/Transform"
version="1.0">
<xsl:output method="text"/>
<xsl:template match="/staff/employee">
employee.<xsl:value-of select="position()"/>.name=<xsl:value-of select="name/text()"/>
employee.<xsl:value-of select="position()"/>.salary=<xsl:value-of select="salary/text()"/>
employee.<xsl:value-of select="position()"/>.hiredate=<xsl:value-of select="hiredate/@year"/>-<xsl:value-of select="hiredate/@month"/>-<xsl:value-of select="hiredate/@day"/>
</xsl:template>
</xsl:stylesheet>
//File: employee.dat
Carl Cracker|75000.0|1987|12|15
Harry Hacker|50000.0|1989|10|1
Tony Tester|40000.0|1990|3|15
Related examples in the same category