Entry validation: Logical Name
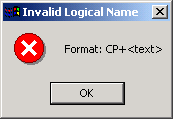
#Pmw copyright
#Copyright 1997-1999 Telstra Corporation Limited, Australia
#Copyright 2000-2002 Really Good Software Pty Ltd, Australia
#Permission is hereby granted, free of charge, to any person obtaining a copy
#of this software and associated documentation files (the "Software"), to deal
#in the Software without restriction, including without limitation the rights
#to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
#copies of the Software, and to permit persons to whom the Software is furnished
#to do so, subject to the following conditions:
#The above copyright notice and this permission notice shall be included in all
#copies or substantial portions of the Software.
#THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
#INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A
#PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
#HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
#OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
#SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
import string
from Tkinter import *
from tkMessageBox import askquestion, showerror
def validLName(value):
valid = 0
try:
if len(value) >= 3:
ucFTC = string.upper(value[:2])
if ucFTC == 'CP':
valid = 1
except:
pass
if valid:
retval = 'CP' + value[2:]
replace = 1
else:
showerror(title='Invalid Logical Name',
message='Format: CP+<text>')
retval = value
replace = 0
return (retval, replace, valid)
class EntryValidation:
def __init__(self, master):
self._ignoreEvent = 0
self._ipAddrV = self._crdprtV = self._lnameV = ''
frame = Frame(master)
Label(frame, text=' ').grid(row=0, column=0,sticky=W)
Label(frame, text=' ').grid(row=0, column=3,sticky=W)
self._lname = self.createField(frame, width=20, row=2, col=2,
label='Logical Name:', valid=self.validate, enter=self.activate)
self._wDict = {self._lname: ('_lnameV', validLName) }
frame.pack(side=TOP, padx=15, pady=15)
def createField(self, master, label='', text='', width=1,
valid=None, enter=None, row=0, col=0):
Label(master, text=label).grid(row=row, column=col-1, sticky=W)
id = Entry(master, text=text, width=width, takefocus=1)
id.bind('<Any-Leave>', valid)
id.bind('<FocusOut>', valid)
id.bind('<Return>', enter)
id.grid(row=row, column=col, sticky=W)
return id
def activate(self, event):
print '<Return>: value is', event.widget.get()
def validate(self, event):
if self._ignoreEvent:
self._ignoreEvent = 0
else:
currentValue = event.widget.get()
if currentValue:
var, validator = self._wDict[event.widget]
nValue, replace, valid = validator(currentValue)
if replace:
self._ignoreEvent = 1
setattr(self, var, nValue)
event.widget.delete(0, END)
event.widget.insert(0, nValue)
if not valid:
self._ignoreEvent = 1
event.widget.focus_set()
root = Tk()
root.option_add('*Font', 'Verdana 10 bold')
root.option_add('*Entry.Font', 'Courier 10')
root.title('Entry Validation')
top = EntryValidation(root)
quit = Button(root, text='Quit', command=root.destroy)
quit.pack(side = 'bottom')
root.mainloop()
Related examples in the same category