Use Entry widgets directly and layout by rows
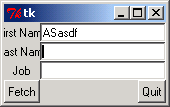
from Tkinter import *
from tkMessageBox import askokcancel
class Quitter(Frame):
def __init__(self, parent=None):
Frame.__init__(self, parent)
self.pack()
widget = Button(self, text='Quit', command=self.quit)
widget.pack(expand=YES, fill=BOTH, side=LEFT)
def quit(self):
ans = askokcancel('Verify exit', "Really quit?")
if ans: Frame.quit(self)
fields = 'First Name', 'Last Name', 'Job'
def fetch(entries):
for entry in entries:
print 'Input => "%s"' % entry.get()
def makeform(root, fields):
entries = []
for field in fields:
row = Frame(root)
lab = Label(row, width=5, text=field)
ent = Entry(row)
row.pack(side=TOP, fill=X)
lab.pack(side=LEFT)
ent.pack(side=RIGHT, expand=YES, fill=X)
entries.append(ent)
return entries
if __name__ == '__main__':
root = Tk()
ents = makeform(root, fields)
root.bind('<Return>', (lambda event, e=ents: fetch(e)))
Button(root, text='Fetch',
command=(lambda e=ents: fetch(e))).pack(side=LEFT)
Quitter(root).pack(side=RIGHT)
root.mainloop()
Related examples in the same category