DOM Operation
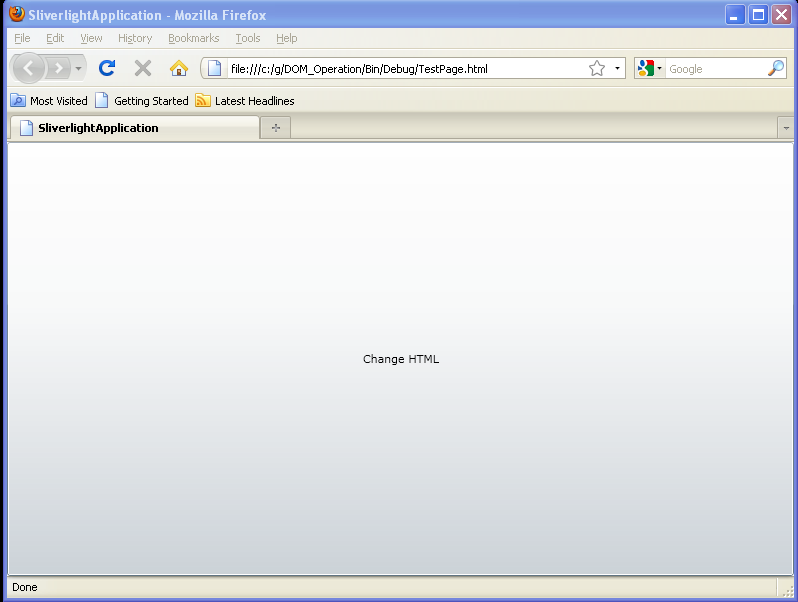
<UserControl x:Class='SilverlightApplication3.MainPage'
xmlns='http://schemas.microsoft.com/winfx/2006/xaml/presentation'
xmlns:x='http://schemas.microsoft.com/winfx/2006/xaml'
xmlns:d='http://schemas.microsoft.com/expression/blend/2008'
xmlns:mc='http://schemas.openxmlformats.org/markup-compatibility/2006'
mc:Ignorable='d'
d:DesignWidth='640'
d:DesignHeight='480'
Loaded="UserControl_Loaded">
<Grid x:Name="LayoutRoot" Background="White">
<Button x:Name="btnChangeHTML"
Content="Change HTML"
Click="btnChangeHTML_Click"/>
</Grid>
</UserControl>
//File: Page.xaml.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
using System.Windows.Browser;
namespace SilverlightApplication3
{
public partial class MainPage : UserControl
{
public MainPage()
{
InitializeComponent();
}
HtmlDocument htmlDocument;
private void UserControl_Loaded(object sender, RoutedEventArgs e)
{
this.htmlDocument = HtmlPage.Document;
HtmlElement btnAlterDOM = htmlDocument.GetElementById("btnAlterDOM");
btnAlterDOM.AttachEvent("onclick", new EventHandler<HtmlEventArgs>(this.OnAlterDOMClicked));
}
public void OnAlterDOMClicked(object sender, HtmlEventArgs e)
{
ScriptObjectCollection h1Collection = htmlDocument.GetElementsByTagName("H1");
HtmlElement element = htmlDocument.CreateElement("input");
element.SetAttribute("type", "text");
element.SetProperty("value", "test");
((HtmlElement)h1Collection[2]).AppendChild(element);
}
private void btnChangeHTML_Click(object sender, RoutedEventArgs e)
{
HtmlElement inputName = htmlDocument.GetElementById("txtNameInput");
HtmlElement btnGetGreeting = htmlDocument.GetElementById("btnGetGreeting");
HtmlElement greeting = htmlDocument.GetElementById("txtGreeting");
inputName.SetStyleAttribute("fontSize", "20px");
greeting.SetStyleAttribute("backgroundColor", "blue");
ScriptObjectCollection h1Collection = htmlDocument.GetElementsByTagName("H1");
foreach (HtmlElement element in h1Collection)
{
element.SetStyleAttribute("backgroundColor", "yellow");
}
bool success = btnGetGreeting.AttachEvent("onclick",new EventHandler<HtmlEventArgs>(this.OnGetGreetingClicked));
}
public void OnGetGreetingClicked(object sender, HtmlEventArgs e)
{
HtmlElement inputName = htmlDocument.GetElementById("txtNameInput");
string nameValue = inputName.GetProperty("Value").ToString();
HtmlElement greeting = htmlDocument.GetElementById("txtGreeting");
greeting.SetProperty("Value", "Good Night " + nameValue);
}
}
}
Related examples in the same category