Displaying and resizing an image
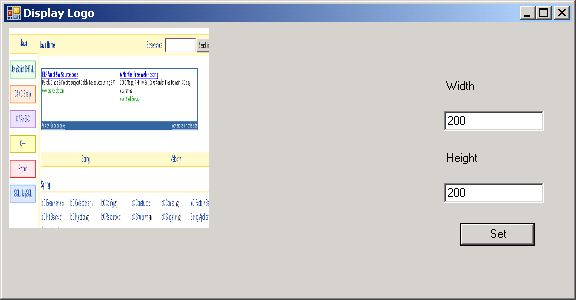
Imports System
Imports System.Drawing
Imports System.Drawing.Drawing2D
Imports System.Windows.Forms
Imports System.Drawing.Text
Public Class MainClass
Shared Sub Main()
Dim myform As Form = New FrmDisplayLogo()
Application.Run(myform)
End Sub ' Main
End Class
Public Class FrmDisplayLogo
Inherits System.Windows.Forms.Form
' width controls
Friend WithEvents txtWidth As TextBox
Friend WithEvents lblWidth As Label
' height controls
Friend WithEvents lblHeight As Label
Friend WithEvents txtHeight As TextBox
Private mGraphicsObject As Graphics
Private mImage As Image
' sets member variables on form load
Private Sub FrmDisplayLogo_Load(ByVal sender As _
System.Object, ByVal e As System.EventArgs) _
Handles MyBase.Load
' get Form's graphics object
mGraphicsObject = Me.CreateGraphics
' load image
mImage = Image.FromFile("figure2.bmp")
End Sub ' FrmDisplayLogo_Load
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
' This call is required by Windows Form Designer.
InitializeComponent()
' Add any initialization after InitializeComponent() call
End Sub ' new
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
Friend WithEvents cmdSetButton As System.Windows.Forms.Button
'Required by the Windows Form Designer
Private components As System.ComponentModel.Container
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.lblWidth = New System.Windows.Forms.Label()
Me.lblHeight = New System.Windows.Forms.Label()
Me.txtWidth = New System.Windows.Forms.TextBox()
Me.txtHeight = New System.Windows.Forms.TextBox()
Me.cmdSetButton = New System.Windows.Forms.Button()
Me.SuspendLayout()
'
'lblWidth
'
Me.lblWidth.Location = New System.Drawing.Point(440, 56)
Me.lblWidth.Name = "lblWidth"
Me.lblWidth.TabIndex = 0
Me.lblWidth.Text = "Width"
'
'lblHeight
'
Me.lblHeight.Location = New System.Drawing.Point(440, 128)
Me.lblHeight.Name = "lblHeight"
Me.lblHeight.TabIndex = 2
Me.lblHeight.Text = "Height"
'
'txtWidth
'
Me.txtWidth.Location = New System.Drawing.Point(440, 88)
Me.txtWidth.Name = "txtWidth"
Me.txtWidth.TabIndex = 1
Me.txtWidth.Text = ""
'
'txtHeight
'
Me.txtHeight.Location = New System.Drawing.Point(440, 160)
Me.txtHeight.Name = "txtHeight"
Me.txtHeight.TabIndex = 3
Me.txtHeight.Text = ""
'
'cmdSetButton
'
Me.cmdSetButton.Location = New System.Drawing.Point(456, 200)
Me.cmdSetButton.Name = "cmdSetButton"
Me.cmdSetButton.TabIndex = 4
Me.cmdSetButton.Text = "Set"
'
'FrmDisplayLogo
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(568, 273)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.cmdSetButton, Me.txtHeight, Me.lblHeight, Me.txtWidth, Me.lblWidth})
Me.Name = "FrmDisplayLogo"
Me.Text = "Display Logo"
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub cmdSetButton_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles cmdSetButton.Click
Dim width As Integer = Convert.ToInt32(txtWidth.Text)
Dim height As Integer = Convert.ToInt32(txtHeight.Text)
If (width > 375 OrElse height > 225) Then
MessageBox.Show("Height or Width too large")
Return
End If
mGraphicsObject.Clear(Me.BackColor)
mGraphicsObject.DrawImage(mImage, 5, 5, width, height)
End Sub
End Class
Related examples in the same category