Declaring, allocating and initializing arrays
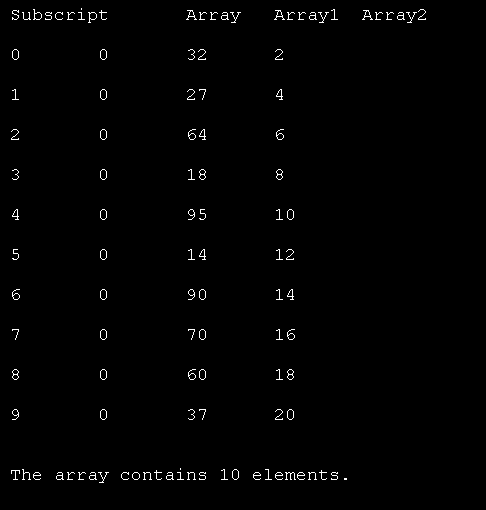
Imports System
Public Class MainClass
Shared Sub Main(ByVal args As String())
Dim i As Integer
Dim array As Integer() ' declare array variable
Dim array1, array2 As Integer() ' declare two arrays
array = New Integer(9) {} ' allocate memory for array
' initializer list specifies number of elements
' and value of each element
array1 = New Integer() {32, 27, 64, 18, 95, _
14, 90, 70, 60, 37}
' allocate array2 based on length of array1
array2 = New Integer(array1.GetUpperBound(0)) {}
' set values in array2 by a calculation
For i = 0 To array2.GetUpperBound(0)
array2(i) = 2 + 2 * i
Next
Console.WriteLine( "Subscript " & vbTab & "Array" & vbTab & _
"Array1" & vbTab & "Array2" & vbCrLf )
' display values in array
For i = 0 To array.GetUpperBound(0)
Console.WriteLine( i & vbTab & array(i) & vbTab & _
array1(i) & vbTab & array2(i) & vbCrLf )
Next
Console.WriteLine( vbCrLf & "The array contains " & _
array.Length & " elements." )
End Sub
End Class
Related examples in the same category
1. | Create elements from one array to another array | | |
2. | Creates a one-dimensional Array of the specified Type and length, with zero-based indexing. | | |
3. | Creates a two-dimensional Array of the specified Type and dimension lengths, with zero-based indexing. | | |
4. | Creates a three-dimensional Array of the specified Type and dimension lengths, with zero-based indexing. | | |
5. | Creates a multidimensional Array of the specified Type and dimension lengths, with zero-based indexing. | | |
6. | Creates a multidimensional Array of the specified Type and dimension lengths, with the specified lower bounds. | | |
7. | Bounded array Example | |  |
8. | For Each loops through Array | | 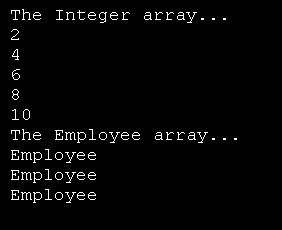 |
9. | Two ways to loop through Array | | 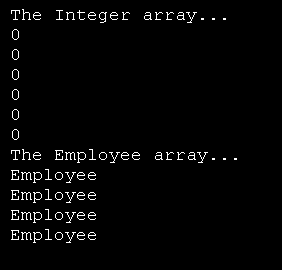 |
10. | A simple class to store in the array | | |
11. | Array UBound | |  |
12. | Set Array Element Value by Index | | |
13. | Array IndexOf and LastIndexOf | |  |
14. | Use Array CreateInstance to Create Array | |  |
15. | Array Performance Test: One-dimensional array | | 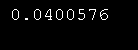 |
16. | Free array's memory | | |
17. | Array Performance Test: SetValue(i, i) | | 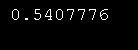 |
18. | Use For Each/Next to find a minimum grade | |  |
19. | Reference an Element in an Array by Index | |  |
20. | Array Upper Bound and Lower Bound | | 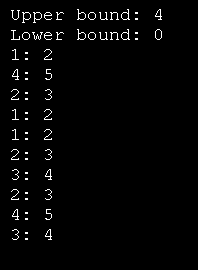 |
21. | Init an Array in Declaration | | 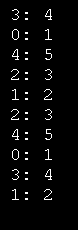 |
22. | Array Length Property | |  |
23. | Declare an Array and reference its member | |  |
24. | Store your won Class in an Array | |  |
25. | Array Class provides methods for creating, manipulating, searching, and sorting arrays | | |
26. | Copies the last two elements from the Object array to the integer array | | |
27. | Creates and initializes a new three-dimensional Array of type Int32. | | |
28. | Sets a range of elements in the Array to zero, to false, or to Nothing, depending on the element type. | | |
29. | Creates a shallow copy of the Array. | | |
30. | Converts an array of one type to an array of another type. | | |
31. | Copies a range of elements from an Array to another Array | | |
32. | Copies all the elements from one Array to another Array | | |
33. | Performs the specified action on each element of the specified array. | | |
34. | Returns an IEnumerator for the Array. | | |
35. | Reverses the sequence of the elements in the entire one-dimensional Array. | | |
36. | Array.GetLength | | |
37. | Gets the lower bound of the specified dimension in the Array. | | |
38. | Uses GetLowerBound and GetUpperBound in the for loop | | |
39. | Gets value at the specified position in the one-dimensional Array | | |
40. | Reverses the elements in a range | | |