Hashtable: add,get,count, Dictionary Key and value Enumerator
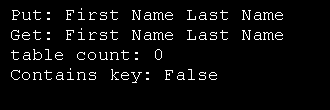
Imports System
Imports System.Collections
Public Class MainClass
Public Shared Sub Main()
Dim table As Hashtable = New Hashtable()
Dim employee As New Employee("First Name", "Last Name")
Try
table.Add("Last Name", employee)
Console.WriteLine("Put: " & employee.ToString())
Catch argumentException As ArgumentException
Console.WriteLine(argumentException.ToString())
End Try
Dim result As Object = table("Last Name")
If Not result Is Nothing Then
Console.WriteLine("Get: " & result.ToString())
Else
Console.WriteLine("Get: " & "Last Name" & " not in table")
End If
table.Remove("Last Name")
Console.WriteLine("table count: " & table.Count)
Console.WriteLine("Contains key: " & table.ContainsKey("Last Name"))
table.Clear()
Dim enumerator As IDictionaryEnumerator = table.GetEnumerator()
While enumerator.MoveNext()
Console.WriteLine(Convert.ToString(enumerator.Value))
End While
enumerator = table.GetEnumerator()
While enumerator.MoveNext()
Console.WriteLine(enumerator.Key )
End While
End Sub
End Class
Public Class Employee
Private firstName, lastName As String
Public Sub New(ByVal first As String, ByVal last As String)
firstName = first
lastName = last
End Sub
Public Overrides Function ToString() As String
Return firstName & " " & lastName
End Function
End Class
Related examples in the same category