IComparer Demo: custom sorting
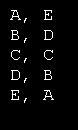
Imports System
Imports System.Collections
Public Class MainClass
Shared Sub Main(ByVal args As String())
Dim students(4) As Student
students(0) = New Student("A", "E")
students(1) = New Student("B", "D")
students(2) = New Student("C", "C")
students(3) = New Student("D", "B")
students(4) = New Student("E", "A")
' Make a comparer.
Dim student_comparer As New StudentComparer
' Sort.
Array.Sort(students, student_comparer)
' Display the results.
Dim txt As String = ""
For i As Integer = 0 To students.GetUpperBound(0)
Console.WriteLine( students(i).ToString() )
Next i
End Sub
End Class
Public Class Student
Public FirstName As String
Public LastName As String
Public Sub New(ByVal first_name As String, ByVal last_name As String)
FirstName = first_name
LastName = last_name
End Sub
Public Overrides Function ToString() As String
Return LastName & ", " & FirstName
End Function
End Class
Public Class StudentComparer
Implements IComparer
Public Function Compare(ByVal x As Object, ByVal y As Object) As Integer _
Implements System.Collections.IComparer.Compare
Dim student1 As Student = DirectCast(x, Student)
Dim student2 As Student = DirectCast(y, Student)
Return String.Compare(student1.ToString, student2.ToString)
End Function
End Class
Related examples in the same category