Quick Sort Demo
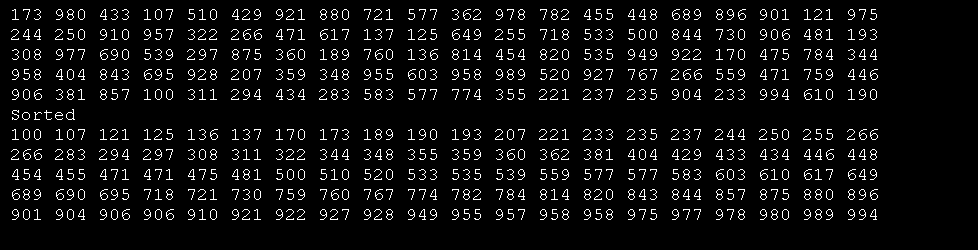
Imports System
Public Class MainClass
Private Shared Values() As Integer
Private Const NUM_VALUES As Integer = 99
Shared Sub Main(ByVal args As String())
Dim random_number As New Random
Dim i As Integer
ReDim Values(NUM_VALUES)
For i = 0 To NUM_VALUES
Values(i) = random_number.Next(100, 1000)
Console.Write( Format$(Values(i)) & " " )
Next i
Console.WriteLine( "Sorted" )
' Sort the numbers.
Quicksort(Values, 0, NUM_VALUES)
For i = 0 To NUM_VALUES
Console.Write( Format$(Values(i)) & " " )
Next i
End Sub
' "Ready-to-Run Visual Basic Algorithms"
' (http://www.vb-helper.com/vba.htm).
Shared Public Sub Quicksort(ByVal list() As Integer, ByVal min As Integer, ByVal max As Integer)
Dim random_number As New Random
Dim med_value As Integer
Dim hi As Integer
Dim lo As Integer
Dim i As Integer
' If min >= max, the list contains 0 or 1 items so
' it is sorted.
If min >= max Then Exit Sub
' Pick the dividing value.
i = random_number.Next(min, max + 1)
med_value = list(i)
' Swap it to the front.
list(i) = list(min)
lo = min
hi = max
Do
' Look down from hi for a value < med_value.
Do While list(hi) >= med_value
hi = hi - 1
If hi <= lo Then Exit Do
Loop
If hi <= lo Then
list(lo) = med_value
Exit Do
End If
' Swap the lo and hi values.
list(lo) = list(hi)
' Look up from lo for a value >= med_value.
lo = lo + 1
Do While list(lo) < med_value
lo = lo + 1
If lo >= hi Then Exit Do
Loop
If lo >= hi Then
lo = hi
list(hi) = med_value
Exit Do
End If
' Swap the lo and hi values.
list(hi) = list(lo)
Loop
' Sort the two sublists.
Quicksort(list, min, lo - 1)
Quicksort(list, lo + 1, max)
End Sub
End Class
Related examples in the same category