Stack Demo: push, pop and peek
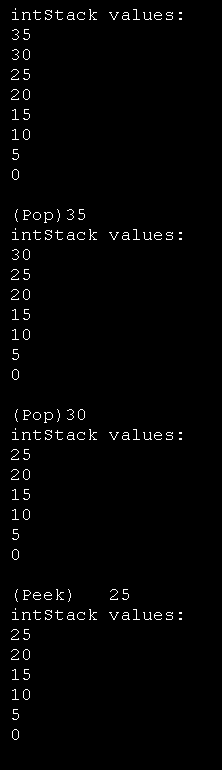
Imports System
Imports System.Collections
Public Class MainClass
Shared Sub Main()
Dim intStack As New Stack( )
'populate the stack
Dim i As Integer
For i = 0 To 7
intStack.Push((i * 5))
Next i
Console.WriteLine("intStack values:")
DisplayValues(intStack)
Console.WriteLine("(Pop){0}", intStack.Pop( ))
Console.WriteLine("intStack values:")
DisplayValues(intStack)
Console.WriteLine("(Pop){0}", intStack.Pop( ))
Console.WriteLine("intStack values:")
DisplayValues(intStack)
Console.WriteLine("(Peek) {0}", intStack.Peek( ))
Console.WriteLine("intStack values:")
DisplayValues(intStack)
End Sub
Public Shared Sub DisplayValues(ByVal myCollection As IEnumerable)
Dim myEnumerator As IEnumerator = myCollection.GetEnumerator( )
While myEnumerator.MoveNext( )
Console.WriteLine("{0} ", myEnumerator.Current)
End While
Console.WriteLine( )
End Sub
End Class
Related examples in the same category