1. | String Object: =, Is and Mid | |  |
2. | String InStr | |  |
3. | String Insert | |  |
4. | Use the Mid function to replace within the string | |  |
5. | Use String.Equals methods | | 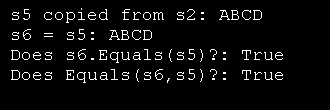 |
6. | Two ways to copy strings | | 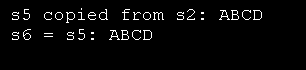 |
7. | Two ways to concatenate strings | |  |
8. | Sub string with only one parameter | | |
9. | String PadLeft | | 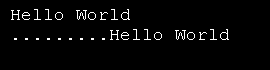 |
10. | String Constructor | | 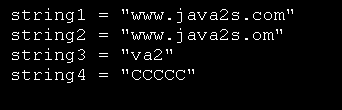 |
11. | String properties Length and Chars and method CopyTo of class String | | 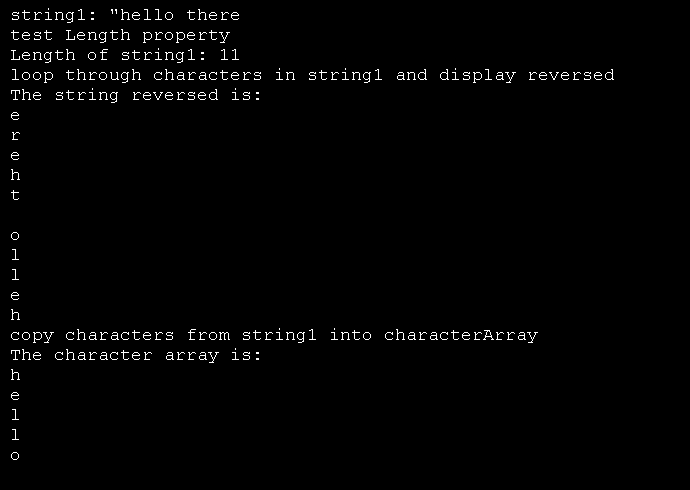 |
12. | Comparing strings | | 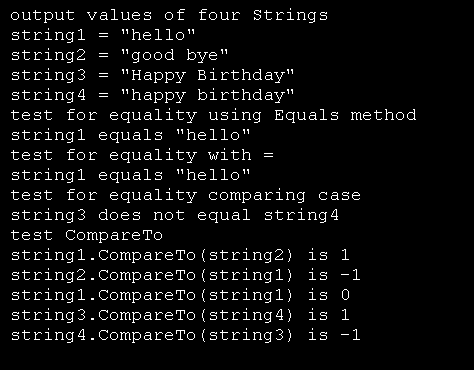 |
13. | Demonstrating StartsWith and EndsWith methods | | 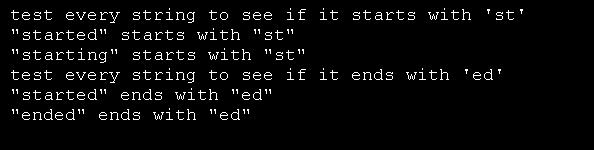 |
14. | String Substring method | |  |
15. | String class Concat method | |  |
16. | String ToLower and ToUpper | | 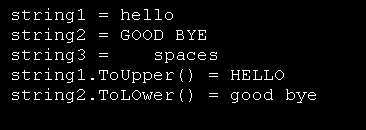 |
17. | String Trim method | | 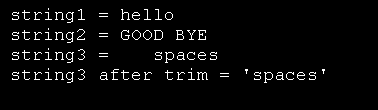 |
18. | String ToString method | | 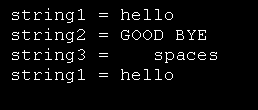 |
19. | String ToCharArray method | | 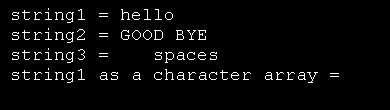 |
20. | Concatenate the strings and display the results | | 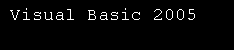 |
21. | The length of the string | |  |
22. | Display the first part of a string | | |
23. | String: set value and display | | |
24. | Concatenate the strings | | 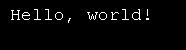 |
25. | Display the length of the string | | 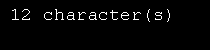 |
26. | Get sub string | |  |
27. | Replace the string occurance | | 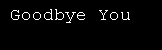 |
28. | String Replace Demo | | 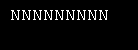 |
29. | Concatenate String with Integer into one String | |  |
30. | String Copy | | 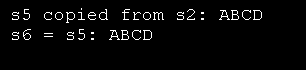 |
31. | String.Equals Demo | | 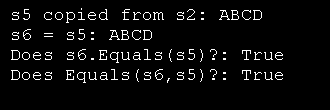 |
32. | Convert to Upper case and Lower Case | | 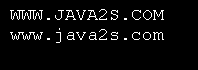 |
33. | Trim String space | |  |
34. | String ends with | |  |
35. | String concatenation | | |
36. | Create a string that consists of a character repeated 20 times | | |
37. | Append Date string to existing string | | |
38. | Extract the second word | | |
39. | String Class Represents text as a series of Unicode characters. | | |
40. | Create a string from a character array | | |
41. | Create a string that consists of a character repeated 20 times | | |
42. | Format Date with D | | |
43. | Append string together | | |
44. | Format a DateTime | | |
45. | Is Surrogate Pair | | |
46. | Is Punctuation and Is WhiteSpace | | |
47. | Create StreamWriter for Unicode encoding | | |
48. | Format Date by different Culture | | |
49. | Parse String to Date with various Culture settings | | |
50. | Perform a word sort using the current (en-US) culture | | |
51. | Perform a word sort using the invariant culture | | |
52. | Perform an ordinal sort | | |
53. | Perform a string sort using the current culture | | |
54. | Find string with different StringComparison | | |
55. | Culture-sensitive test for equality | | |
56. | Ordinal test for equality | | |
57. | String.Format Method (IFormatProvider, String, Object[]) | | |
58. | Format Date with different Culture | | |
59. | StringBuilder Class Represents a mutable string of characters | | |
60. | CultureInfo Class provides information about a specific culture | | |
61. | CultureInfo's English Name and | | |
62. | CultureInfo.CurrentCulture Property gets the CultureInfo that represents the culture used by the current thread. | | |
63. | Encoding Class represents a character encoding. | | |
64. | String.Chars Property gets the Char object at a specified position in the current String object. | | |
65. | String.Concat | | |
66. | String.Contains Method returns a value indicating whether the specified String object occurs within this string. | | |
67. | String.Copy | | 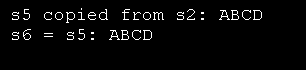 |
68. | String.CopyTo Method | | |
69. | String.EndsWith (String, StringComparison) | | |
70. | String.Equals Method | | |
71. | String.Equals (String) | | |
72. | String.Format (IFormatProvider, String, Object[]) | | |
73. | Customer Formatter | | |
74. | String.Format Method (String, Object) | | |
75. | String.Format (String, Object, Object) | | |
76. | String.GetEnumerator | | |
77. | String.GetHashCode Method | | |
78. | String.GetTypeCode Method returns the TypeCode for class String. | | |
79. | Sample for String.Intern(String) | | |
80. | String.IsNullOrEmpty | | |
81. | String.Join Method | | |
82. | Concatenates the members of a collection, using the specified separator between each member. | | |
83. | String.Length Property gets the number of characters in the current String object. | | |
84. | String.PadLeft Method (Int32) | | |
85. | String.PadLeft Method (Int32, Char) | | |
86. | String.PadRight | | |
87. | String.PadRight Method (Int32, Char) | | |
88. | String.Remove | | |
89. | Deletes a specified number of characters from this instance beginning at a specified position. | | |
90. | String.Replace (Char, Char) | | |
91. | String.Replace (String, String) | | |
92. | Retrieves a substring | | |
93. | Retrieves a substring which starts at a specified character position and has a specified length. | | |
94. | Copies the characters to a Unicode character array. | | |
95. | Convert to lowercase according to Culture settings | | |
96. | Convert string to lowercase | | |
97. | Convert String to lowercase using the casing rules of the invariant culture. | | |
98. | [Object].ReferenceEquals | | |
99. | String.ToUpper (CultureInfo) | | |
100. | String.ToUpper | | |
101. | Convert String to uppercase using the casing rules of the invariant culture. | | |
102. | Removes all leading and trailing occurrences of a set of characters | | |
103. | Removes all leading and trailing white-space characters from the current String object. | | |
104. | Removes all trailing occurrences of a set of characters | | |
105. | Encode the original string using the ASCII encoder | | |
106. | CharEnumerator Class supports iterating over a String | | |