Execute two queries together
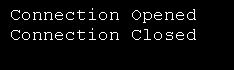
Imports System
Imports System.Data
Imports System.Data.SqlClient
public class MainClass
Shared Sub Main()
Dim thisConnection As New SqlConnection("server=(local)\SQLEXPRESS;" & _
"integrated security=sspi;database=MyDatabase")
'Sql Query 1
Dim sql1 As String ="SELECT FirstName FROM Employee; "
'Sql Query 2
Dim sql2 As String ="SELECT LastName FROM Employee;"
'Combine queries
Dim sql As String = sql1 & sql2
'Create Command object
Dim thisCommand As New SqlCommand _
(sql, thisConnection)
Try
' Open Connection
thisConnection.Open()
Console.WriteLine("Connection Opened")
' Execute Query
Dim thisReader As SqlDataReader = thisCommand.ExecuteReader()
Catch ex As SqlException
' Display error
Console.WriteLine("Error: " & ex.ToString())
Finally
' Close Connection
thisConnection.Close()
Console.WriteLine("Connection Closed")
End Try
End Sub
End Class
Related examples in the same category